Welcome to our exploration of numpy in Python! We are embarking on an exciting expedition into the realm of numerical processing and high-level mathematical functions, all neatly packed into this incredibly useful library.
Impatient to start experimenting with arrays, conjuring up sophisticated computations and diving headfirst into the universe of numerical data manipulation? Let’s embark on this mind-expanding journey together!
Table of contents
What is numpy?
Often hailed as the cornerstone of numerical computing in Python, numpy (Numerical Python) is a library that provides a high-performance multidimensional array object. It is versatile, resourceful and forms the bedrock for a tremendous amount of Python-based scientific computing.
With numpy, you acquire the ability to handle large, multi-dimensional arrays and matrices of numerical data, something Python’s native list struggles with. Equipped with an impressive collection of mathematical functions, numpy lends itself admirably to perform operations on these arrays.
Integrating numpy into your Python arsenal amplifies your data handling capacity, paving the way for you to master critical computational skills. Additionally, for coding enthusiasts who relish optimizing their code’s efficiency or dream of venturing into data-intensive fields such as artificial intelligence, games or scientific computing, getting familiar with numpy is a voyage you should definitely embark on.
The Basics of numpy arrays
We will begin with understanding how to create basic numpy arrays and perform simple operations on it. Let’s create our first numpy array.
import numpy as np a = np.array([1, 2, 3]) print(a)
See, here np.array() is used to create a numpy array. When you run this code, a numpy array of three elements is created. The output should be:
[1 2 3]
Multi-dimensional arrays
Numpy equips us to create multi-dimensional arrays. So let’s create a 2-Dimensional array, which can be thought of as a matrix with rows and columns.
import numpy as np b = np.array([(1,2,3),(4,5,6)]) print(b)
Here we see that we just passed in two tuples into the array function which created the 2D array. The output for this should be:
[[1 2 3] [4 5 6]]
Numpy mathematical operations
Numpy has several mathematical operations that can be performed on arrays. Let’s take a look at the simple add and multiply operations.
import numpy as np a = np.array( [20,30,40,50] ) b = np.array( [1,2,3,4] ) c = a-b d = a*b print(a) print(b) print(c) print(d)
This operation subtracts each element of array ‘b’ from ‘a’ and multiplies each element of ‘a’ with ‘b’. So, the output of array ‘c’ will be ‘a’-‘b’ and ‘d’ will be ‘a’*’b’. Now isn’t that simple and intuitive!
Numpy special functions
There are several functions in numpy such as sine, cosine, exponential, etc. Let’s illustrate this with an example:
import numpy as np import matplotlib.pyplot as plt a = np.arange(0,3*np.pi, 0.1) b = np.sin(a) plt.plot(a,b) plt.show()
Here we have first defined an array ‘a’ which contains values from 0 to 3π. Then, the sine of ‘a’ is computed and the graph is plotted. Therefore, you can use numpy to effectively perform complex mathematical functions as well.
Array computations with numpy
numpy offers a wealth of functions to perform statistical computations across arrays, such as calculating the mean, min, max, etc. Let’s try these functions out:
import numpy as np a = np.array([(1,2,3,4),(3,4,5,6)]) print(a.min()) print(a.max()) print(a.sum())
In this case, the min function gives us the smallest element in the array, max gives the largest element and sum gives us the total of all elements in the array.
Array reshaping and slicing with numpy
numpy gives you a lot of flexibility in reshaping arrays and accessing the elements. Let’s look at how to slice and reshape an array:
import numpy as np a = np.array([(1,2,3,4),(3,4,5,6)]) print('Slicing first row:', a[0]) print('Reshaping to 1D:', a.ravel())
Here, we have used array indexing to slice the first row of the array. The `ravel()` function is used to convert a numpy array to 1D.
Mathematical functions on numpy arrays
Let’s perform mathematical functions like square root and standard deviation on numpy arrays.
import numpy as np a = np.array([(1,2,3,4),(3,4,5,6)]) print('Square root:', np.sqrt(a)) print('Standard deviation:', np.std(a))
As expected, the square root function `sqrt()` gives the square root of each element in the array, and the standard deviation function `std()` does accordingly.
Stacking numpy arrays
A very useful feature of numpy is to vertically or horizontally stack two arrays. Here’s how you do it:
import numpy as np x = np.array([(1,2,3),(3,4,5)]) y = np.array([(1,2,3),(3,4,5)]) print('Vertical stacking:\n', np.vstack((x,y))) print('Horizontal stacking:\n', np.hstack((x,y)))
The output would be the vertical and horizontal stacking of the arrays ‘x’ and ‘y’. This is extremely handy when you want to combine two arrays in a particular way.
Where to go next
Having tested the waters of numpy, aren’t you ready to dive deeper into the vast ocean that is Python? Your next step, dear reader, is to further enhance your skills and enrich your programming journey by exploring our Python Mini-Degree.
The Python Mini-Degree offered by Zenva Academy is a comprehensive, cutting-edge suite of courses that will guide you through the world of Python, right from the basics to more advanced concepts such as algorithms, object-oriented programming, game development, and app development.
Prepare to get hands-on as you learn by coding your own games, building algorithms, and developing real-world apps. Graduate from novice to professional, broadening the horizons of your programming adventure under the guidance of our skilled and experienced instructors who are well-equipped with practical knowledge, industry insights and are certified by Unity Technologies and CompTIA.
On successful completion, you will not only have a robust portfolio comprising your Python projects but also be enriched with the skills needed to carve your path in the Python landscape across various industries.
Already have a handle on Python basics and yearn for more advanced challenges? Sprint towards our Python Courses, specifically curated for intermediate-level learners. Delve deeper into Python and continue expanding your skill-set!
Remember that the key to mastering any programming language is diligent practice and continual learning. We, at Zenva, are always here to guide you and enable you to triumph over your coding challenges. Embrace the adventure and continue your journey with us!
Conclusion
Brimming over with functionality, versatility, and power, numpy invigorates Python with the capability to handle complex and sizable data arrays efficiently. With numpy by your side, you are outfitted to battle all the complexities and challenges that come with numerical computing.
Your journey towards mastering numpy is akin to mining for precious gems – the deeper you dig, the more rewarding the treasures you unearth! Equip your repertoire with this invaluable skill, and unearth its multifaceted potentials by diving into our Python Mini-Degree. Immerse yourself in the vibrant universe of Python, guided by our meticulously crafted courses, and let your knowledge propagate exponentially.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
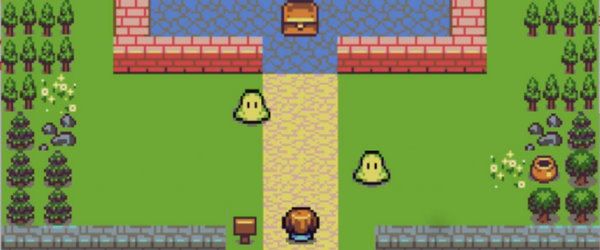
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.