Welcome to this exciting journey into C# (pronounced “C-Sharp”) for Unity! Whether you are looking to develop your first game or trying to incorporate advanced strategies into your existing game development toolkit, this course will guide you every step of the way in an exciting, hands-on fashion.
Table of contents
What is C#?
C# is a type-safe, scalable and object-oriented language, which allows you to create reusable blocks of code. These features make it a fundamental language for game development in Unity, a popular game engine.
C# and Unity: An unbeatable duo
Unity uses C# as one of its primary scripting languages. This allows game developers to handle everything from character behaviors to Artificial Intelligence in games.
Why You Should Learn C# for Unity
C# is not only intuitive but it’s also versatile. It prioritizes simplicity, making it easier for beginners to grasp the basics, yet it remains powerful enough for even the most complex game development tasks.
Apart from this, mastering C# opens up a world of possibilities for game development in Unity. So whether you dream of creating your own game, or want to work with existing game prototypes, Unity and C# knowledge becomes critical.
Fundamentals of C# in Unity
Moving on, let’s dive into some primary C# concepts that you will encounter during your Unity game development process.
Variables and Data Types
In C#, you’ll find yourself constantly using different types of variables. These might include integers, floats, strings, booleans.
int myScore = 150; // integer float mySpeed = 10.5f; // floating point number string playerName = "John Doe"; // string bool isAlive = true; // boolean
Conditional Statements
You use these to control the flow of your code. The ‘if’ statement is one of the most frequently used.
if (isAlive) { Debug.Log("Player is still alive"); } else { Debug.Log("Player has been killed"); }
Loops
Looping structures allow you to repeat blocks of code until a condition is met. Here’s how to implement a simple ‘for’ loop for iterating ten times.
for (int i = 0; i < 10; i++) { Debug.Log("This is loop iteration number: " + i); }
Functions or Methods
Functions, also known as methods, are blocks of code designed to perform specific tasks. They can also return a value.
static void SayHello() { Debug.Log("Hello from Zenva!"); } static int AddTwoNumbers(int number1, int number2) { return number1 + number2; }
Remember, this is just the tip of the iceberg. As you progress with C# and Unity, you’ll encounter many more concepts and habits that will help you to make remarkable games. Start learning and practicing more today with us at Zenva!
More Powerful Tools with C# in Unity
After mastering the basics, there’s a lot more you can do with C#. Let’s continue expanding your knowledge with more advanced concepts.
Classes and Objects
Classes are one of the cornerstones of OOP (Object-oriented programming). They’re a blueprint from which individual objects are created. Each object then has specific properties and behaviors defined in the class.
public class Player { public string name; public int level; public bool isAlive; // Method behavior of a player public void DisplayDetails() { Debug.Log("Player Name: " + name + " Level: " + level + " isAlive: " + isAlive); } }
Once defined, you can create an object:
Player player1 = new Player(); player1.name = "John"; player1.level = 10; player1.isAlive = true; player1.DisplayDetails();
Arrays
Arrays in C# are collections of variables of the same type. They’re useful for storing multiple values in a single data structure.
int[] levelScores = {100, 90, 80, 70, 60}; // Access array element Debug.Log(levelScores[1]); // Output: 90
User Input
User interaction in Unity is captured using C#. For instance, to move a game object based on the arrow keys, we typically use Input.GetKey()
// Inside an Update() method if (Input.GetKey(KeyCode.UpArrow)) { transform.Translate(Vector3.up * speed * Time.deltaTime); }
Exception Handling
When something unexpected happens while your game is running, it throws an exception. C# provides try, catch, and finally blocks to handle these errors gracefully.
try { int result = 10 / 0; // This will throw an exception } catch (DivideByZeroException) { Debug.Log("You cannot divide by zero"); } finally { Debug.Log("End of exception handling"); }
Understanding these advanced C# concepts will greatly enhance your Unity game development skills. The more you explore and practice with us at Zenva, the more powerful and efficient your coding becomes!
Working with Collections: Lists and Dictionaries
While arrays are great, they have their limitations, such as a fixed size. In contrast, Lists in C# are dynamic collections, enabling you to add or remove elements during runtime.
List<int> playerScores = new List<int>(); playerScores.Add(90); // Adding an element playerScores.Remove(90); // Removing an element Debug.Log(playerScores.Contains(90)); // Checks if an element exists
Dictionaries, on the other hand, hold value pairs (keys and values) and are perfect when you need a way to look up values based on a key.
Dictionary playerLevelScores = new Dictionary(); playerLevelScores.Add("Level1", 90); // Adding a key-value pair playerLevelScores.Remove("Level1"); // Removing a key-value pair Debug.Log(playerLevelScores.ContainsKey("Level1")); // Checks if a key exists
Understanding Unity Events
C# in Unity shines when handling game events. For example, the ‘Start’ and ‘Update’ methods are part of the Unity lifecycle and are used commonly across the framework.
void Start() { // This is called once at the beginning of the scene } void Update() { // This is called once per frame }
Similarly, there are other event functions in Unity like Awake(), FixedUpdate(), LateUpdate() which are used based on different scenarios.
Interacting with Unity’s API
C# is the go-to language for interacting with Unity’s API, which is essential for doing anything meaningful in the game engine. Examples include instantiating objects, handling collisions, and managing UI.
Creating an object:
GameObject sphere = GameObject.CreatePrimitive(PrimitiveType.Sphere); sphere.transform.position = new Vector3(0, 0, 0);
Handling collisions:
void OnCollisionEnter(Collision collision) { if (collision.gameObject.name == "Enemy") { Debug.Log("Player hit the enemy"); } }
Managing UI:
public Text scoreText; void Update() { int score = CalculateScore(); // A hypothetical method scoreText.text = "Score: " + score; }
Mastering all these concepts and more will gradually make you a proficient Unity game developer with C#! At Zenva, we’re looking forward to developing your skills and seeing what amazing games you’ll create!
Continuing Your C# Unity Journey
From an absolute beginner to an exceptional game developer, the journey won’t be easy, but it will be worth it. Coding in C#, mastering Unity, and creating compelling games can open up countless opportunities. We, at Zenva, believe that with the right resources and passion, anyone can become proficient in this field.
We encourage you to explore our Unity Game Development Mini-Degree program. This comprehensive collection of courses takes learners through the process of game development using Unity, teaching a wide range of topics such as game mechanics, audio effects, custom game assets, and even creating enemy AI and animation. It’s designed to cater for all skill levels, from beginners venturing into game development for the first time, to seasoned developers looking to sharpen their skills.
Alongside this, we have an expansive collection of courses in our Unity course catalog. Spanning across various focuses within Unity, these courses are an excellent way to deepen your understanding and refine your skill set in specific areas. Keep learning, keep creating, and let’s bring your game development dreams to life!
Conclusion
Our journey into the world of C# for Unity has just begun. The possibilities are as vast as your imagination can stretch. The tools are accessible, the community is supportive, and the potential impact of your creations is profound. Now, it’s up to you to take charge and bring your unique vision to life.
At Zenva, we are always eager to guide passionate learners like you. Our Unity Game Development Mini-Degree program is tailored to turn you from a novice into a professional game developer. Don’t wait – dive in now and let the fun of learning and creating begin!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
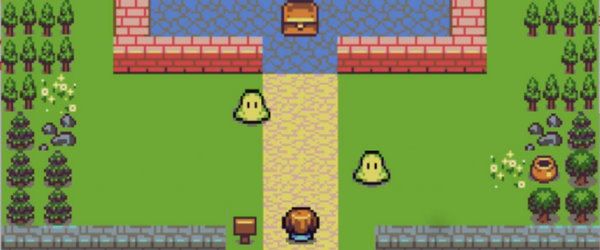
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.