In the world of coding and computer science, one pattern stands among the crowd as a prime example that fuses mathematics with programming: the Fibonacci sequence. By exploring the implementation of this mathematical phenomenon in the C# language, we can create powerful applications and proposals with practical uses in game development.
Table of contents
What is the Fibonacci Sequence?
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. This simple structure has countless applications ranging from financial market analysis to procedural level creation in games.
Why Implement it with C#?
C# is a modern, object-oriented programming language built around the .NET framework. Given its powerful features and easy-to-understand syntax, it becomes a great choice to implement complex mathematical algorithms like the Fibonacci sequence.
What Can it Do in Game Development?
In game development, the Fibonacci sequence can be used to design procedural content, such as tile-based puzzles or generative map layouts. It can give your game a dynamic, unpredictable element, making each playthrough unique, all whilst optimizing your code and giving the player a mathematically balanced game experience.
Calculating the Fibonacci Sequence Using Recursion
The Fibonacci sequence can be achieved through a beneficial feature of several programming languages known as recursion. Recursion is when a function calls itself in its own definition. We can use recursion to calculate the Fibonacci sequence in C# as follows:
public static int fibonacciRecursive(int n) { if (n <= 1) { return n; } else { return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2); } }
The function starts by checking if the input number is less than or equal to 1. If this is true, it returns the input number. If not, it calls itself twice with the (n-1) and (n-2). The returned outcome is the sum of the two preceding numbers, thus establishing the Fibonacci sequence.
Calculating the Fibonacci Sequence Using Iteration
While recursion delivers a clean, easy-to-understand Fibonacci sequence calculation, it’s typically slower and may lead to stack overflow issues on larger inputs. The iterative approach using loops can be faster and more reliable:
public static int fibonacciIterative(int n) { int a = 0; int b = 1; for (int i = 0; i < n; i++) { int temp = a; a = b; b = temp + b; } return a; }
This function initializes two variables, ‘a’ and ‘b’, to represent the two preceding numbers in the Fibonacci sequence. Note that ‘a’ starts at zero and ‘b’ at one – the first two numbers in the sequence. We then use a for-loop to iterate ‘n’ times, each time shifting ‘a’ and ‘b’ one place further along the sequence.
Displaying the Fibonacci Sequence
With our Fibonacci functions created, let’s create a quick program to display the first 10 numbers of the Fibonacci sequence in the console:
for (int i = 0; i < 10; i++) { Console.WriteLine(fibonacciIterative(i)); }
This loop calls our fibonacciIterative function for the first 10 integers, displaying each Fibonacci number on a new line in your console. It’s a simple way to visually inspect that our Fibonacci functions work correctly.
Generating a Fibonacci Series in a List
If we want to generate an entire Fibonacci series up to a certain length, we can do so by simply looping through our Fibonacci function and adding each result to a list:
List<int> fibonacciList = new List<int>(); for (int i = 0; i < 10; i++) { fibonacciList.Add(fibonacciIterative(i)); } foreach (int number in fibonacciList) { Console.WriteLine(number); }
Each iteration of the loop calculates the i-th Fibonacci number and adds it to the list. The foreach loop then prints every number in this list, displaying your Fibonacci series in the console.
Creating a Function that Returns Fibonacci Series
We can encapsulate the previous code snippet into a function that returns a Fibonacci series of any desired length. This function can be used in your larger C# applications. Here’s how it’s done:
public static List<int> GenerateFibonacciSeries(int length) { List<int> fibonacciList = new List<int>(); for (int i = 0; i < length; i++) { fibonacciList.Add(fibonacciIterative(i)); } return fibonacciList; }
This function essentially combines our Fibonacci and list-creation logic into a single, reusable function. You can use this to quickly generate Fibonacci series in your C# programs.
Visualizing the Fibonacci Series in a Game
By implementing the Fibonacci series, we can add dynamic and unpredictable patterns to procedurally generated games. For example, we could use a Fibonacci series to create a spiral pattern in a tile-based puzzle:
public void GenerateSpiral() { List<int> fibonacciList = GenerateFibonacciSeries(10); for (int i = 0; i < fibonacciList.Count; i++) { for (int j = 0; j < fibonacciList[i]; j++) { PlaceTile(i, j); } } }
This script assumes a function `PlaceTile(int x, int y)` that places a game tile at a specific position based on the Fibonacci number. The generated pattern could enhance a puzzle game with varying difficulty, triggered by the step changes in the Fibonacci series. Having such patterns can add a new layer of complexity and variety to your games.
Loop Optimization for Larger Fibonacci Searches
Generating larger Fibonacci numbers can start to perform poorly with the recursive method. Due to this limitation, C# programmers often optimize the loop with memoization – keeping a record of calculated Fibonacci numbers to avoid redundant calculations.
public long GetFibonacci(int n) { long[] fibonacciSequence = new long[n+2]; fibonacciSequence[0] = 0; fibonacciSequence[1] = 1; for (int i = 2; i <= n; i++) { fibonacciSequence[i] = fibonacciSequence[i-1] + fibonacciSequence[i-2]; } return fibonacciSequence[n]; }
Above, we solve the Fibonacci problem with dynamic programming by solving the sub-problems and building up to the final number. This method improves the function’s time complexity to linear, making it far quicker for large inputs. By utilizing optimization techniques like these, we ensure our applications perform to their utmost, and keep our players fully engaged.
Implementing the Fibonacci sequence in C# can open up a wide range of possibilities for game developers, going from enriching procedural contents to create engaging game patterns. Equipped with the resources shared in this tutorial, you’re now ready to give it a try in your own game projects! Happy Coding!
Making Your Fibonacci Efficient for Real World Applications
When you want to find a specific Fibonacci number, it’s worth considering the two previous methods’ computational inefficiency. As the size of ‘n’ grows, the number of recursive calls or iterations required becomes impractical. Thankfully there’s an even more efficient method, rooted in the concept of matrices and power calculations. Here’s how to do it:
public static int FastFibonacci(int n) { int[,] F = new int[,] { { 1, 1 }, { 1, 0 } }; if (n == 0) return 0; power(F, n - 1); return F[0, 0]; } public static void multiply(int[,] F, int[,] M) { int x = F[0, 0] * M[0, 0] + F[0, 1] * M[1, 0]; int y = F[0, 0] * M[0, 1] + F[0, 1] * M[1, 1]; int z = F[1, 0] * M[0, 0] + F[1, 1] * M[1, 0]; int w = F[1, 0] * M[0, 1] + F[1, 1] * M[1, 1]; F[0, 0] = x; F[0, 1] = y; F[1, 0] = z; F[1, 1] = w; } public static void power(int[,] F, int n) { int i; int[,] M = new int[,] { { 1, 1 }, { 1, 0 } }; for (i = 2; i <= n; i++) multiply(F, M); }
This approach comes from the observation that the Fibonacci sequence can be represented as a matrix. The power function significantly reduces the time complexity of finding large Fibonacci numbers.
Finding the Index of a Fibonacci Number
Another question arises when we need to find the index of a particular Fibonacci number. We don’t need to generate the whole sequence to find the index; we can simply use the following formula:
public static int GetIndexOfFibonacciNumber(double FibonacciNumber) { return (int)Math.Floor((Math.Log(FibonacciNumber) * Math.Sqrt(5) + 0.5) / Math.Log((1 + Math.Sqrt(5)) / 2)); }
With this function, you input a number ‘n’ and it returns the index ‘i’ such that F(i) <= n < F(i+1). This can be handy when dynamically generating a Fibonacci sequence in response to the player's actions within gameplay.
Calculating Fibonacci in Parallel
In C#, you can use the power of your CPU cores to calculate the Fibonacci sequence in parallel, significantly speeding up the calculation for large values.
public static BigInteger[] FibonacciParallel(int n) { if (n = 2) { numbers[1] = 1; } Parallel.For(2, n, i => { numbers[i] = numbers[i - 1] + numbers[i - 2]; }); return numbers; }
The FibonacciParallel function creates and calculates an array of the first ‘n’ Fibonacci numbers. The actual calculation in the Parallel.For loop is done using our usual Fibonacci formula.
Final Closing
Understanding how to implement the Fibonacci sequence in C# can lead you to other fascinating avenues in both game development and beyond. You can leverage this sequence for procedural content generation, game balancing, NPC AI logic, and many other areas.
Implementing these strategies into your C# games offers a unique challenge for veteran game developers and beginners alike. We at Zenva hope that our exploration of the Fibonacci sequence in game development has inspired you to continue innovating and adding unique, dynamic elements to your games. The only limit is your imagination! Happy Coding!
Where to Go Next – The Journey Continues
With a solid understanding of implementing the Fibonacci sequence in C#, you’ve just scratched the surface of what’s possible in game development. It’s time to kick it up a notch and dive deeper into the world of creating your own games.
We recommend you check out our comprehensive Unity Game Development Mini-Degree. This course encompasses a range of topics such as game mechanics, cinematic cutscenes, audio effects, and much more. Built around Unity, one of the most popular game engines globally, this Mini-Degree offers both beginners and experienced developers the perfect opportunity to enhance their skills and versatility.
Also, for those wanting a broader collection, we suggest you explore our Unity courses. Your journey doesn’t end here; with Zenva, you can go from beginner to professional, entering a world packed with imaginative possibilities.
Keep pushing the boundaries, keep creating, and keep learning – the path to mastery is one of continuous exploration. At Zenva, we’re thrilled to be part of that journey with you. Happy Coding!
Conclusion
From understanding what the Fibonacci sequence is, to implementing it in C#, and even exploring its applications in game development, we dove deep into a novel realm where mathematics and programming intertwine. By mastering this timeless sequence, you open the doors to dynamic gameplay elements, optimized code, and a fresh perspective on game design.
We at Zenva hope this exploration has not only enhanced your coding skills but sparked a thrill for continuous learning. Remember, this is just the tip of the iceberg, and there’s so much more to explore in the vast universe of coding and game creation. Keep the momentum going and dive into our game-changing Unity Game Development Mini-Degree journey. Happy Coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
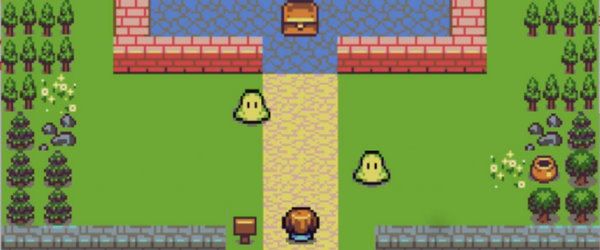
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.