Welcome to your starting point in the world of GDScript! If you are an aspiring game developer or simply a coding enthusiast looking to add another language to your programming palette, you’re in the right place. This self-contained tutorial aims to equip you with the basics of GDScript, a high-level, dynamically typed scripting language tailor made for the Godot game engine.
Table of contents
What is GDScript?
GDScript is a language designed specifically for Godot, an open-source game engine known for its user-friendly and versatile features. Modeled closely after Python, GDScript is not just accessible to beginners, but also offers robust functionality for seasoned coders.
What is it for?
GDScript allows you to control every aspect of your game logic. From character movement and enemy AI behavior to weather systems and procedural generation, almost anything that makes a game interactive can be programmed using GDScript.
Why should I learn it?
Understanding GDScript is key if you want to unlock the potential of the Godot game engine. Since GDScript is designed specifically for Godot, the two are tightly integrated, which enhances performance and ease-of-use. Furthermore, if you are already familiar with Python or similar scripting languages, GDScript will feel quite familiar and intuitive.
Setting up your First GDScript Module
Your first step in GDScript is to create a new script. This is the basic shell where your code will live and run from. Here’s how it looks:
extends Node func _ready(): pass
In this script, “extends Node” is defining the class that your script is extending. The “_ready()” function is a placeholder and will be called when your script first runs.
Variables and Constants
Creating variables in GDScript is simple. You use the `var` keyword, and constants are defined with the `const` keyword. Unlike many languages, you don’t need to specify a type (although you can) as GDScript is dynamically-typed. Here are some examples:
var health = 100 const MAX_HEALTH = 200 var player_name = "Player 1"
Conditionals and Control Flow Structures
GDScript supports the standard if, else and elif control structures that many other languages use.
if health <= 0: print("Game Over") elif health == MAX_HEALTH: print("Full Health") else: print("Health: " + str(health))
It also supports for and while loops:
for i in range(0, 10): print(i) var count = 0 while count < 5: print(count) count += 1
Functions
Functions in GDScript are defined using the `func` keyword. Functions can take arguments and optionally return a value. Here’s an example of a tiny function that returns the square of a number:
func square(num): return num * num
And here we call the function:
print(square(5)) # prints 25
Now you’ve covered all the fundamentals, the essential building blocks of GDScript, including script setup, variables, conditions, loops, and functions. Feel free to experiment with these concepts and create your own sequences to get a better handle on them.
Collections – Arrays and Dictionaries
GDScript includes Collection types to store multiple values together. The primary types are Arrays, akin to lists in Python, and Dictionaries, similar to maps or hash tables in other languages.
Creating an Array is as simple as listing the elements in square brackets:
var players = ["John", "Mark", "Emily", "Anna"] print(players[0]) # prints "John"
You can append to an Array:
players.append("Steve") print(players) # prints ["John", "Mark", "Emily", "Anna", "Steve"]
And you can even have an Array of Arrays to create a multi-dimensional array:
var matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] print(matrix[2][1]) # prints 8
Dictionaries, on the other hand, consist of pairs of keys and values. They allow for fast lookups based on keys.
var player_ages = {"John": 17, "Mark": 25, "Emily": 22} print(player_ages["Mark"]) # prints 25
With dictionaries, you can easily add, delete, and modify values:
player_ages["Anna"] = 23 # adds a new key-value pair del player_ages["John"] # deletes the John's entry player_ages["Emily"] = 23 # modifies Emily's age to 23
Object-Oriented Programming
As an object-oriented language, GDScript supports the creation of custom classes and inheritance. Let’s create a Player class with some basic properties:
class Player: var name var health var position func _init(name, health, position): self.name = name self.health = health self.position = position
Then we can create instances:
var player1 = Player.new("John", 100, Vector2(0, 0)) var player2 = Player.new("Mark", 200, Vector2(50, 50))
And access their properties:
print(player1.name) # prints "John" print(player1.health) # prints 100
Inheriting from another class is done using the `extends` keyword:
class Warrior extends Player: var strength func _init(name, health, position, strength): .name = name .health = health .position = position self.strength = strength
With concepts like collections and object-oriented programming under your belt, you’re well on your way to creating complex and dynamic scripts in GDScript. Dive deeper, experiment, and don’t hesitate to build upon these foundations.
Loading and Managing Scenes
In Godot, levels, menus or screens inside a game are referred to as scenes. GDScript provides a convenient way to manage and change scenes.
To load a scene into memory, you use the `load()` function:
var main_scene = load("res://main_scene.tscn")
Once a scene has been loaded, it can be instantiated to create a tree of nodes that can be added to the current scene:
var main_instance = main_scene.instance() get_tree().root.add_child(main_instance)
In the above code, `get_tree().root` returns the root of the active scene tree.
Interacting with the filesystem (taking in user data, reading and writing savegame files, etc.) is also a crucial part of almost any game. GDScript provides a simple, built-in way to read from and write to files using the `File` class.
To write to a file:
var file = File.new() file.open("user://data.dat", file.WRITE) file.store_string("Some user data") file.close()
To read from a file:
var file = File.new() file.open("user://data.dat", file.READ) var data = file.get_as_text() file.close() print(data) # prints "Some user data"
GDScript also offers various inbuilt functions for mathematical operations and manipulations. Here’s how you can perform a linear interpolation between two numbers:
var start = 10 var end = 20 var weight = 0.5 var result = lerp(start, end, weight) print(result) # prints 15.0
Godot’s Vector types have a lot of useful methods. You can normalize a Vector2 (i.e., change it to have a length/magnitude of 1):
var vector = Vector2(20, 30) vector = vector.normalized() print(vector.length()) # prints 1.0
Remember, keep experimenting with various GDScript features, explore its deep integration with the Godot engine, and you’re sure to master creating captivating and interactive games in no time!
Where to Go Next?
The journey of learning and mastering GDScript doesn’t end here. There’s so much more to explore and experiment with in the dynamic world of game development. We at Zenva offer numerous courses, from beginner to professional, covering programming, game development, and AI. Over 250 supported courses are available to boost your career as you learn coding, create games, and earn valuable certificates.
We particularly recommend our Godot Game Development Mini-Degree, which is an extensive set of interactive courses focusing on building cross-platform games with the popular, lightweight, and open-source Godot engine.
This amazing compilation covers using 2D and 3D assets, GDScript programming language, gameplay control flow, player and enemy combat, item collection, and UI systems. You will get to work on projects from various genres, allowing you to build a solid portfolio of real Godot projects while gaining skills crucial for a career in game development.
Moreover, for a wider array of learning options, do check out our collection of Godot Courses.
Thus, continue your learning journey with Zenva, where you can evolve from a beginner to a professional game developer at your own pace and convenience.
Conclusion
With the help of this tutorial, you’ve taken your first steps onto your GDScript learning path. As you’ve seen, GDScript isn’t just an accessible and intuitive language, but also a mighty tool that allows you to leverage the full-power of the Godot game engine. The more you play with it, the more you’ll realize its potential in creating interactive, fun, and memorable games.
Why wait? It’s time to turn those fantastic game ideas in your head into reality! Embark on your journey to mastering GDScript and Godot with our comprehensive Godot Game Development Mini-Degree. Whether you’re a beginner just starting out or a seasoned developer looking to broaden your skillset, Zenva is here to guide you every step of the way. Let’s code, create, and conquer the gaming world together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
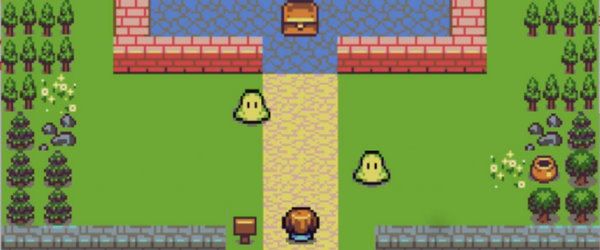
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.