Welcome to this exciting tutorial on the GDScript class constructor. As one of the fundamental aspects of the popular GDScript language used in Godot game development, it’s a concept that is both intriguing and pragmatic in shaping your programming journey. In the world of game creation, a class constructor helps you mould your gaming characters and their behaviours, making it an invaluable tool in your developer’s toolkit.
Table of contents
What is the GDScript Class Constructor?
In GDScript language, a class constructor is essentially a special method in a class that is automatically called when an instance of the class is created. It’s the foundational framework that defines and initializes the properties of an object.
Why Learn About the GDScript Class Constructor?
Understanding how to leverage the power of the class constructor in GDScript can open endless possibilities in game development. This knowledge allows you to:
- Define dynamic game elements
- Refine character behaviour
- Streamline code and improve your game’s performance
This tutorial will reveal the magic behind the scenes of GDScript class constructor, equipping you with not only the essential understanding but also the ability to apply this knowledge in practical game development scenarios.
Remember, every great gamer was once a beginner. No matter where you are in your coding journey, this tutorial is designed to guide you through mastering the GDScript class constructor in an engaging, straightforward and comprehensive manner.
So, let’s roll up our coding sleeves and dive right in!
GDScript Class Constructor: Basic Syntax
The simplicity and easy-to-understand syntax in GDScript makes it unique and ideal for beginners to quickly grasp core concepts.
Let’s look at our initial example of the basic structure of a class constructor:
class ClassName: var property func _init(): property = value
Creating a Class Constructor
Creating a class constructor in GDScript is quite simple and is done through the _init() method. Let’s create a constructor for a class called Character.
class Character: var name func _init(): name = "Unknown"
In the example above, we have a Character class with a constructor. The constructor initializes the name of the character to “Unknown”.
Passing Parameters to the Constructor
In GDScript, you can pass parameters to the constructor in order to initialize class properties. This enhances the flexibility and dynamism in your game development.
class Character: var name func _init(character_name): name = character_name
In the code snippet above, we have a Character class where the constructor takes a parameter character_name. This parameter is used to initialize the name of the character.
Creating Object Instances
After creating your class and defining the constructor, the next step is to create an instance of the class. This is done by simply calling the class.
var newCharacter = Character.new("John")
Here, we create a new instance of the Character class and pass “John” as an argument to the constructor. The name of the newCharacter object will be set to “John”.
Conclusion
As we have seen, the GDScript class constructor is an essential aspect of object-oriented programming in the world of game development. It allows you to define and initialize the properties that your gaming characters and objects will have, essentially laying the foundational blueprint for your game’s behavior and performance.
At Zenva, our role is to simplify these concepts for you, breaking down complex ideas into easily understandable modules that equip you with the skills needed in the demanding field of game development.
Delving Deeper: Exploring Advanced Uses of Class Constructor
Now that we have a basic understanding of the GDScript class constructor, let’s move ahead and explore some advanced uses and practical applications in game development.
Multiple Parameters on Initialization
GDScript class constructor can accept multiple parameters. This can be useful when you’re creating more complex game characters or objects. Let’s create a class for a game character that requires a name and an age:
class Character: var name var age func _init(character_name, character_age): name = character_name age = character_age
We can now create an instance of the Character class with these two parameters:
var newCharacter = Character.new("John", 25)
Parameter Defaults
You can also set default values for the parameters in GDScript class constructors. These will be used if no value is passed when an instance is created:
class Character: var name var age func _init(character_name = "Unknown", character_age = 18): name = character_name age = character_age
If you create a new character without specifying the name and age:
var newCharacter = Character.new()
The character’s name will be “Unknown” and the age will be 18.
Calling Parent Class Constructor
When working with inheritance in GDScript, you might need to call the parent class’s constructor. You can do this using the .new() method, followed by the .(super).init() function:
class Character: var name func _init(character_name): name = character_name class Wizard(Character): var magic_level func _init(character_name, magic_level): .(super)._init(character_name) self.magic_level = magic_level
Here, we’ve used inheritance to create a subclass of ‘Character’ called ‘Wizard’. In the ‘Wizard’ class, we’ve called the parent class’s constructor to initialize the name of the wizard. We’ve then initialized a new wizard-specific property, ‘magic_level’.
As a GDScript developer and an eager game creator, these advanced uses of the class constructor will enhance your ability to add depth and complexity to your games. Class constructors are indeed a vital cog in GDScript game development, enabling you to orchestrate outstanding gaming experiences within Godot’s realm.
At Zenva, we’re dedicated to producing content that educates, engages, and empowers you to reach new heights in your game development journey.
Enhancing Game Objects with Class Constructors
Now that we’re well-versed with the basics and some of the advanced features of the GDScript class constructor let’s put it to work and enhance our game characters.
Creating Game Characters with Unique Attributes
In a game, you may have characters with unique attributes that perform different roles. Let’s define a class for a Rogue character, a specialist character type in many RPG games.
class Rogue: var name var agility var stealth func _init(rogue_name, rogue_agility, rogue_stealth): name = rogue_name agility = rogue_agility stealth = rogue_stealth
By defining different parameters in our class constructors, we can create characters that are unique and offer different gameplay experiences.
Skipping Instance Property Initialization
In some cases, you may want to create an instance of a class without initializing every property. A clever way to achieve this is by providing default values in our class constructor:
class Rogue: var name var agility = 5 # default value var stealth = 5 # default value func _init(rogue_name): name = rogue_name
Thus, creating an instance of a Rogue class would require specifying just the name:
var newRogue = Rogue.new("Shadow")
Agility and stealth will automatically default to 5 for the new rogue, Shadow.
Updating Instance Properties
Our game may require the ability to update certain attributes of a character. This can be easily achieved by defining getter and setter methods in our class:
class Rogue: var name var agility = 5 # default value var stealth = 5 # default value func _init(rogue_name): name = rogue_name func set_agility(new_agility): agility = new_agility func get_agility(): return agility
We can now update our rogue’s agility like so:
var newRogue = Rogue.new("Shadow") newRogue.set_agility(10)
Class Constructors and pygame
If you’re familiar with pygame, you might have noticed some similarities with GDScript and how class constructors are used. Taking this knowledge to pygame, you might see something like:
class Player(): def __init__(self, startx, starty): self.x = startx self.y = starty
In both GDScript and Python (and by extension, pygame), class constructors play a massive role in defining and initializing our game objects.
Enjoy incorporating these techniques into your game development repertoire. The marvel of class constructors is their ability to efficiently engineer your game’s behavioral framework, adding depth and functionality.
Continue to explore, learn, and innovate with us at Zenva, your trusty partner in your game development journey. Empowered with these skills, you can create more dynamic game characters and rock the Godot Engine!
Fuel Your Learning Journey
Your dedication to understanding GDScript class constructors and their application in Godot game development is commendable. However, this is just the tip of the iceberg when it comes to the expansive universe of game creation.
At Zenva, we invite you to fuel your desire to learn more and advance your skills even further. Our range of immersive courses, from beginner to professional levels, include Python programming, game development, and artificial intelligence. You can collect knowledge, create games, and earn certificates that reflect your expanding skillset.
We highly recommend you explore our Godot Game Development Mini-Degree. It’s a comprehensive program that guides enthusiasts like you in building cross-platform games using the powerful Godot 4 engine. The series provides an in-depth understanding of 2D and 3D assets, GDScript, gameplay control flow, combat dynamics, item collection, UI systems, and more. With coverage from the foundational levels of Godot 4 to advanced platformers, RPGs, RTS, and survival games, this curriculum caters to both beginners and experienced developers.
Our vast collection of Godot Courses is also a valuable resource to diversify your learning journey. Remember, continuous learning is the key to unlocking the most thrilling possibilities in game development.
With Zenva, every step you take accelerates you from a beginner coder to a proficient game creator. So, keep learning, keep creating, and let’s redefine gaming together!
Conclusion
Decoding GDScript class constructors and wielding their power in your game development adventures can dramatically revolutionize the gaming experiences you create. Not only does this foundational concept help you breathe life into your game characters, but it also paves the way for fine-tuned control and customization of your gaming universe.
As you continue on this thrilling journey of creation with us at Zenva, remember to explore our Godot Game Development Mini-Degree. It’s a treasure chest filled with all the knowledge, tips, and tools you need to create impressive and immersive games. Let’s conquer the captivating world of game development together, one class constructor at a time!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
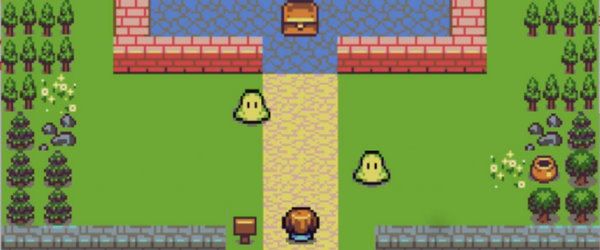
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.