From games and mobile apps, to operating systems and software platforms, Object-Oriented Programming (OOP) is an essential skill for any programmer. Think of what you can do by manipulating and controlling your own user-designed tools, instead of being limited by the rigid structure of procedural programming.
Table of contents
What is OOP in C#?
OOP is a programming paradigm based on the concept of ‘objects’ rather than logic. These ‘objects’ are instances of classes, which are user-defined data structures consisting of fields (variables) and methods (functions). When we talk about OOP with C#, we are referring to the use of C# language constructs in creating these objects and using them to write efficient and maintainable code. Unlike procedural programming, which is linear, OOP allows programs to be organized around objects and data, rather than logic and actions.
Why Learn OOP in C#?
In the world of game design and software development, OOP is key. Here’s why:
- Code Reusability: Classes created for an object can be reused in different programs, reducing redundancy.
- Code Maintenance: It’s easier to manage and modify code when it’s distributed into class-based objects.
- Security: Data inside classes (the objects) can be made private, so can only be manipulated and accessed through class methods.
Encapsulation, Inheritance, and Polymorphism are just a few of the essential concepts in OOP that can make your code more efficient and easier to manage. In the following sections, we will delve into writing code in C#, exploring best practices in establishing classes, objects, methods, and properties. All this, while keeping your code clean and your programming workflow smooth.
Classes and Objects in C#
In C#, everything is contained within a class. A class is a blueprint for creating objects. An object is an instance of a class. Let’s start by creating a basic class in C#:
public class Animal { //... }
This example creates an empty class called Animal. Now, let’s create an object from this class:
Animal myAnimal = new Animal();
In the above code, we create a new instance of our Animal class, called myAnimal.
Fields and Methods in C#
Fields are variables that are declared directly within a class. Methods are operations that objects can perform.
Let’s add some fields and methods to our Animal class:
public class Animal { public string name; // a field public void Speak() { // a method Console.WriteLine("The animal speaks!"); } }
Fields and methods can be accessed using the dot (.) operator:
Animal myAnimal = new Animal(); myAnimal.name = "Lion"; myAnimal.Speak(); //prints "The animal speaks!"
Constructors in C#
A constructor in C# is a special method used to initialize objects. The constructor has the same name as the class:
public class Animal { public Animal() { //a constructor Console.WriteLine("An animal has been created."); } }
This constructor will be called each time an object is created:
Animal myAnimal = new Animal(); //prints "An animal has been created."
Properties in C#
Properties are a way to control the access levels of class data. They combine the characteristics of both fields and methods.
Let’s add a property to our Animal class:
public class Animal { private string name; // a private field public string Name { // a property get { return name; } set { name = value; } } }
Now, let’s use the Name property:
Animal myAnimal = new Animal(); myAnimal.Name = "Lion"; Console.WriteLine(myAnimal.Name); //prints "Lion"
We created a ‘Name’ property that allows us to access the ‘name’ field. The ‘get’ accessor returns the field’s value, while the ‘set’ accessor assigns a value to the field.
Inheritance in C#
Inheritance is a cornerstone of OOP, where one class can inherit fields and methods from another. Let’s use inheritance to create a more specific type of Animal:
public class Mammal : Animal { public void ShowParent() { Console.WriteLine("I'm a mammal and I'm also an animal."); } }
In this example, the Mammal class is inheriting the field and methods of the Animal class. To use:
Mammal myMammal = new Mammal(); myMammal.ShowParent(); myMammal.Name = "Elephant"; Console.WriteLine(myMammal.Name);
Polymorphism in C#
Polymorphism is the ability of an object to take on many forms. There are two types of polymorphism in C# – static and dynamic. Static polymorphism is achieved with method overloading. Dynamic polymorphism with method overriding:
Method Overloading
Overloading allows methods in the same class to have the same name but different parameters:
public class Animal { public void Speak() { Console.WriteLine("The animal speaks!"); } public void Speak(string sound) { Console.WriteLine("The animal makes a sound: " + sound); } }
Animal myAnimal = new Animal(); myAnimal.Speak(); //prints "The animal speaks!" myAnimal.Speak("Roar"); //prints "The animal makes a sound: Roar"
Method Overriding
Overriding allows a subclass to provide a specific implementation of a method already provided by its parent class:
public class Animal { public virtual void Speak() { Console.WriteLine("The animal speaks!"); } } public class Lion : Animal { public override void Speak() { Console.WriteLine("The lion roars!"); } }
Lion myLion = new Lion(); myLion.Speak(); //prints "The lion roars!"
This way, the Speak() method in the Lion class will be invoked over the Speak() method in the Animal class.
Abstraction and Encapsulation in C#
Abstraction lets us hide complexities by providing only fundamental functionality. Encapsulation confines data manipulation within the class:
public class Animal { private string name; //encapsulation public string Name { //abstraction get { return name; } private set { name = value; } //encapsulation } public Animal(string name) { Name = name; } }
Animal myAnimal = new Animal("Lion"); Console.WriteLine(myAnimal.Name); //prints "Lion"
Encapsulation and abstraction allow us to maintain a clear separation between class definitions and how objects interact with each other, thereby making our code more secure and maintainable.
Working with Interfaces in C#
Interfaces allow us to define a contract for what a class should do, without specifying how it should do it. This brings in a higher level of abstraction to our code:
public interface IAnimal { void Speak(); } public class Cat : IAnimal { public void Speak() { Console.WriteLine("The cat meows!"); } } public class Dog : IAnimal { public void Speak() { Console.WriteLine("The dog barks!"); } }
IAnimal myCat = new Cat(); myCat.Speak(); //prints "The cat meows!" IAnimal myDog = new Dog(); myDog.Speak(); //prints "The dog barks!"
Here, both Cat and Dog implement the IAnimal interface, which makes sure they both have a Speak() method.
Working with Enums in C#
Enums (short for enumerations) are used when we want a variable to only have certain predefined constants. Enum enhances code readability and reduces errors caused by invalid values:
public enum AnimalType { Mammal, Bird, Fish }
AnimalType myAnimal = AnimalType.Mammal; Console.WriteLine(myAnimal); // prints "Mammal"
An enum is a value type, and the default underlying type of the enumeration elements is int.
Exception Handling in C#
Exceptions represent errors that occur during application execution. In C#, exception handling is done using four keywords: try, catch, finally and throw:
try { int result = 10 / 0; } catch (DivideByZeroException e) { Console.WriteLine("Can't divide by zero!"); Console.WriteLine(e.Message); } finally { Console.WriteLine("This line executes no matter what."); }
The code within the try block is protected code that may cause an exception. The catch block contains the code that handles the exception. The finally block contains the cleanup code that is executed regardless of what happens in the try catch blocks.
All these concepts – OOP, interfaces, enums, and exception handling, are essential in the journey to mastering C#. With these skills, you not only weave seamless narratives in the gaming world, but also create dynamic and robust software platforms. Practice them, and stay tuned for more interesting guides from us!
Next Steps in Your Programming Journey
Having discovered the essentials of Object-Oriented Programming in C#, you’re in an exciting place right now. You are enabled to design your own game mechanics, build software applications and even define operating systems – the world of code, quite literally, is at your fingertips.
But don’t stop there! The exciting part of learning to code is that there is always more to learn, more to create. We at Zenva highly recommend continuing your learning journey with our Unity Game Development Mini-Degree. This comprehensive collection of courses covers various programming paradigms including game mechanics, audio effects, and more, taught across multiple programming languages. You’ll get to build your own Unity games, perfect for incorporating all the C# skills you’ve learned here.
Are you keen on exploring more Unity-related content? Head to our Unity collection. Here at Zenva, we offer over 250 supported courses that cater to both beginners and professionals, helping you continue your journey in becoming a proficient programmer and game developer. The interesting journey of coding awaits – happy learning!
Conclusion
Mastering Object-Oriented Programming with C# is just the beginning of your adventure into the creative realm of game development and software programming. It’s a world filled with endless possibilities and infinite creativity. Don’t stop here! Dive deeper into the ocean of coding with our comprehensive courses at Zenva. We inspire learners like you to transform their worlds with technology, and we would be thrilled to be a part of your learning journey.
Embark upon the next phase of this exciting journey with our Unity Game Development Mini-Degree and continue expanding your knowledge. Remember, in the field of technology and programming, the key to success is continuous learning. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
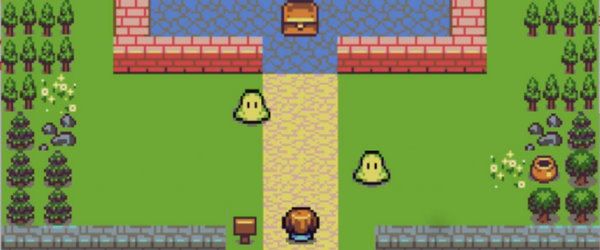
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.