Delving into the world of programming, understanding the mechanisms that turn your code into action is crucial. Among these mechanisms, the concepts of compilers and interpreters stand as pillars of code execution. Grasping their differences, functionalities, and use cases not only adds to your foundational knowledge but also enhances your ability to choose the right tools and approaches for your projects.
Whether you are starting out on your programming adventures or looking to consolidate your knowledge, this exploration of compilers and interpreters is intended to demystify these concepts, making them accessible and exciting. Join us as we embark on a journey through the world of code execution, and discover how understanding these tools can unleash the full potential of your coding prowess.
Table of contents
What is a Compiler?
A compiler is a powerful tool that transforms the code written in a high-level programming language into machine code that a computer’s processor can execute. It does this through several stages including parsing, compiling, and linking.
What is an Interpreter?
On the flip side, an interpreter translates and executes code “on the fly,” which means it reads, analyzes, and processes each line of code one by one, without the need to compile it into machine code beforehand.
What Are They For?
Both compilers and interpreters serve a similar purpose – to turn human-readable code into something a machine can understand and execute. However, they approach this task in different ways, each suitable for different scenarios and types of programming languages.
Why Should I Learn About Them?
Knowing the difference between compilers and interpreters will enable you to make informed decisions about which programming languages and tools to use for your projects. It’s vital for troubleshooting, optimizing performance, and understanding the execution of your code at a fundamental level.
Working with Compilers
Compilers are an integral part of the development process, especially when working with statically typed languages like C, C++, and Java. Let’s begin by exploring some basic compilation examples in different programming languages. These will serve as a primer on how to transform your source code into executable programs.
Compiling a Simple C Program
// hello.c #include int main() { printf("Hello, World!\n"); return 0; }
To compile the C program above, you would use a C compiler such as gcc:
gcc hello.c -o hello
This command compiles ‘hello.c’ into an executable file named ‘hello’.
Compiling a C++ Program
C++ is another language typically requiring compilation. Here’s a basic example:
// hello.cpp #include int main() { std::cout << "Hello, World!" << std::endl; return 0; }
To compile this with a g++ compiler, you would issue:
g++ hello.cpp -o hello
You’ll get an executable ‘hello’ that you can run to display “Hello, World!”.
Compiling Java Code
Java source files are first compiled into bytecode, which the Java Virtual Machine (JVM) can then interpret and execute. The Java Development Kit (JDK) includes the necessary compiler called ‘javac’.
// HelloWorld.java public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Compilation of a Java program is accomplished with the ‘javac’ command:
javac HelloWorld.java
This results in a ‘HelloWorld.class’ file containing the bytecode.
Understanding Interpreters
Interpreters provide us with the means to execute scripts and programs directly, which is common in languages such as Python, Ruby, and JavaScript. Below, we examine some of the basic usages of interpreters.
Running Python Scripts
Python uses an interpreter to execute scripts written in .py files. Here’s a simple script:
# hello.py print("Hello, World!")
To run this script, use the Python interpreter:
python hello.py
The output “Hello, World!” is displayed immediately.
Executing JavaScript with Node.js
Node.js allows us to run JavaScript on the server-side. Here’s a snippet to log “Hello, World!” to the console:
// hello.js console.log('Hello, World!');
To execute this with Node.js, enter:
node hello.js
This will print “Hello, World!” in your terminal or command prompt.
Running Ruby Code
Ruby scripts are executed in a manner similar to Python scripts. Consider the following Ruby code:
# hello.rb puts "Hello, World!"
The corresponding command to run this script with the Ruby interpreter is:
ruby hello.rb
The output in the terminal will be “Hello, World!”.
By understanding how to use both compilers and interpreters with these basic examples, you’re taking your first steps into a broader world of programming. These foundational skills are crucial and will pave the way for more complex programming tasks ahead.Let’s delve deeper into the practical use of compilers and interpreters by exploring more complex examples and scenarios that you might encounter in your coding journey.
Advanced Compilation and Interpretation Examples
Compiling Multiple C Files
When working with larger projects in C, you’re likely to have multiple source files. Here’s how you can compile them together:
// main.c #include "hello.h" int main() { say_hello(); return 0; } // hello.c #include #include "hello.h" void say_hello() { printf("Hello, multi-file world!\n"); } // hello.h #ifndef HELLO_H #define HELLO_H void say_hello(); #endif
To compile these files into a single executable, you would use:
gcc main.c hello.c -o hello_program
This produces ‘hello_program’, an executable that runs your program.
Conditional Compilation with the C Preprocessor
The C preprocessor allows for conditional compilation. This can be used to compile code selectively:
// main.c #include #define DEBUG 1 int main() { #ifdef DEBUG printf("Debug mode is on.\n"); #else printf("Debug mode is off.\n"); #endif return 0; }
Compiling and running this will output the message “Debug mode is on.” If you remove or comment out ‘#define DEBUG 1’ and recompile, it’ll say “Debug mode is off.”
Overloading Functions in C++
C++ supports function overloading, where multiple functions have the same name but different parameters. The c++ compiler distinguishes which function to call based on the parameters used:
// main.cpp #include void display(int num) { std::cout << "Displaying int: " << num << std::endl; } void display(double num) { std::cout << "Displaying double: " << num << std::endl; } int main() { display(5); // Calls the int version display(5.5); // Calls the double version return 0; }
Compiling with ‘g++ main.cpp -o display’ creates an executable that demonstrates function overloading.
Compiling Java for Different Versions
Java allows you to specify the version of bytecode to generate, aiding compatibility with different versions of the JVM:
// HelloWorld.java public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, Java version!"); } }
To compile for a specific JVM version, you might use:
javac -source 1.8 -target 1.8 HelloWorld.java
This ensures compatibility with Java 8 environments.
Using the Interactive Python Shell
Python’s interpreter also offers an interactive mode, where you can write and execute Python code in real-time. Simply invoking ‘python’ without any arguments will enter this mode:
python
You can then type Python code directly into the console:
>>> print("Hello, Interactive Python!") Hello, Interactive Python!
Automating Tasks with Shell Scripting
Shell scripts are interpreted and can automate tasks on Unix-like systems. Here is an example of a Bash script that prints system information:
#!/bin/bash echo "Current directory:" pwd echo "System uptime:" uptime
You can run this script by assigning execute permissions and invoking it directly:
chmod +x script.sh ./script.sh
The ‘echo’ and ‘uptime’ commands will execute sequentially, displaying the current directory and system uptime.
Understanding these more nuanced examples of how compilers and interpreters work provides a deeper insight into how your code comes to life. It highlights the adaptability of these tools across various programming scenarios, strengthening your capability to handle diverse programming challenges.Continuing with our journey into compilers and interpreters, let’s explore further with additional code examples that can enrich your understanding and fluency in utilizing these indispensable tools in programming.
We’ll look at scenarios involving complex conditions, integrating external libraries, and branching out into handling user input—all of which are common tasks when developing software.
Integrating External Libraries in C
Using external libraries is a common need. For instance, you might want to incorporate the ‘math’ library in C to perform complex mathematical operations.
// math_example.c #include #include int main() { double result = sqrt(49.0); printf("The square root of 49 is %f\n", result); return 0; }
Compile with the math library linked:
gcc math_example.c -lm -o math_example
The ‘-lm’ flag tells the gcc compiler to link against the ‘math’ library.
Creating Templated Functions in C++
Templates allow for generic programming. Here’s how you might create a templated function in C++ to work with various data types:
// template_example.cpp #include template T findMax(T a, T b) { return (a > b) ? a : b; } int main() { std::cout << "Max of 2 and 5 is " << findMax(2, 5) << std::endl; std::cout << "Max of 2.2 and 5.5 is " << findMax(2.2, 5.5) << std::endl; return 0; }
Compile using ‘g++’:
g++ template_example.cpp -o template_example
This program will find and display the maximum of two numbers, for both integer and floating-point types.
Java User Input Example
Handling user input is essential for interactive applications. Here’s how you can read from the console in Java:
// UserInput.java import java.util.Scanner; public class UserInput { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter your name: "); String name = scanner.nextLine(); System.out.println("Hello, " + name + "!"); scanner.close(); } }
Compile and run the Java code:
javac UserInput.java java UserInput
The program will prompt the user to enter their name and greet them accordingly.
Python External Module Usage
Python is renowned for its extensive library ecosystem. Here’s how to use an external library, like ‘requests’, to make an HTTP request:
# request_example.py import requests response = requests.get('https://api.github.com') print(response.status_code)
First, ensure you have the ‘requests’ module installed:
pip install requests
Then run the Python script:
python request_example.py
The script outputs the status code of the HTTP response.
JavaScript Async/Await Example
Modern JavaScript uses async/await for handling asynchronous operations. Here’s a Node.js script that reads a file asynchronously:
// async_example.js const fs = require('fs').promises; async function readFile(filePath) { try { const data = await fs.readFile(filePath, 'utf8'); console.log(data); } catch (error) { console.error('Error reading file:', error); } } readFile('example.txt');
Run the script with Node.js:
node async_example.js
If ‘example.txt’ exists, it will print its contents; otherwise, it will display an error message.
These examples highlight the power and flexibility of compilers and interpreters across various languages and scenarios. Whether you’re dealing with external libraries, asynchronous operations, or user interaction, understanding how to effectively utilize these tools can significantly elevate the quality and efficiency of your code. Keep experimenting with these examples to deepen your practical understanding, and you’ll be on your way to mastery in no time.
Continuing Your Learning Journey
The world of programming is vast and ever-evolving, and the knowledge you’ve gained about compilers and interpreters is a valuable stepping stone on your learning path. To further hone your skills and continue to grow as a developer, immersing yourself in the practical world of coding is imperative. One excellent way to do this is by exploring Python Mini-Degree on Zenva Academy, which provides a thorough introduction to Python programming—one of the most versatile and in-demand languages in the industry.
Through our Python Mini-Degree, you’ll delve into diverse topics, create your own projects, and gain the practical experience necessary to excel in the field. Python serves as a great language for both beginners and experienced developers due to its readability and broad application in different domains. And if you’re seeking even more, our extensive catalogue of Programming Courses offers content across a range of topics to satisfy the learning needs at any stage of your career.
Join our community of learners at Zenva and let us support your journey to becoming a proficient developer. Whether you are starting from scratch or building on existing knowledge, we have the resources to guide you from beginner to professional. So, take the next step, and start writing your own code story today!
Conclusion
We hope this exploration into compilers and interpreters has ignited a curiosity and deeper understanding of the mechanisms behind code execution. Remember, the knowledge you’ve gained is more than theoretical; it’s a practical toolkit that will serve you in deciphering the intricacies of programming languages and their environments. As you continue to build and refine your expertise, let Zenva Academy be your guide. With our Python Mini-Degree, you’re not just learning to code, you’re crafting a skillset that will open doors to endless possibilities in the tech industry.
Harness the full potential of your newfound understanding and join the ranks of successful developers who started their journey with us. Whether you’re iterating through your first loop or deploying a full-stack application, Zenva’s commitment to your growth remains unwavering. So don’t hesitate—embark on this continuous learning adventure, and let’s code a better future together.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
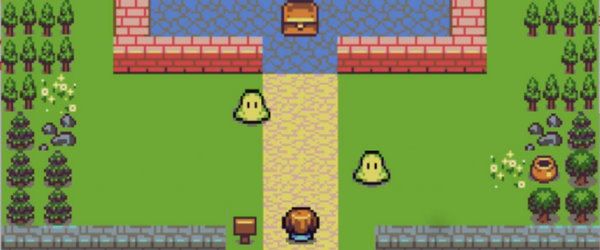
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.