Diving into the realm of programming, one can’t help but encounter the term “primitive data types” early on in their learning journey. Understanding these fundamental ingredients is essential to master the craft of coding, just as a chef must understand their spices. Whether you’re new to the domain or brushing up your skills, a robust grasp of these basic building blocks will serve you in writing efficient and effective code that stands strong at its core. So what are primitive data types, and why should you invest your time in learning them? Join us as we embark on an exploration of these critical components in programming, delivered through engaging examples and analogies. Ready to add another tool to your coding toolkit? Let’s get started!
Table of contents
What Are Primitive Data Types?
In programming, primitive data types are the simplest form of data that a language provides. They are the basic types of data upon which all other more complex data structures are built. Think of them as the atoms in the world of coding – small, essential, and the foundation for constructing anything more substantial.
What Are They Used For?
Primitive data types serve as the backbone for storing and manipulating data within your programs. Without these, you wouldn’t be able to hold onto simple pieces of information like numbers, characters, or even true and false values. They’re the starting point for any variable creation and value manipulation in your code.
Why Should I Learn About Them?
Having a solid understanding of primitive data types is akin to learning the alphabet before writing a novel. It’s fundamental as it will:
– Equip you with the knowledge to declare variables and handle data efficiently.
– Aid in debugging by understanding type-related errors.
– Prepare your foundation for learning more complex data structures and algorithms.
– Enhance your ability to communicate with other programmers and read code more effectively.
There might be various programming languages out there, but the concept of primitive data types is universal, making this knowledge transferable and invaluable as you continue your coding endeavors.
Common Primitive Data Types in Programming
In most programming languages, you’ll encounter a similar set of primitive data types. These include integers, floating-point numbers, characters, and booleans. Let’s take a closer look at each of these through some examples.
Integers: Represent whole numbers without a decimal point. They can be positive or negative and are used for counting, indexing, and more.
int age = 25; int temperature = -10; int numberOfStudents = 42;
Floating-point Numbers: Used for representing numbers with decimal points. They come in handy for calculations requiring fractions or precision beyond integers.
float interestRate = 4.5f; double pi = 3.141592653589793; double gravitationalConstant = 6.67430e-11;
Characters: A single letter, number, symbol, or whitespace. Enclosed within single quotes, characters form the basis for text processing.
char initial = 'S'; char digit = '7'; char symbol = '$';
Booleans: This primitive data type has only two possible values: true or false. Booleans are essential for control flow in programming through conditions and loops.
boolean isRaining = true; boolean isLeapYear = false; boolean hasPassedTest = true;
As you delve into specific programming languages, these examples may vary slightly in syntax but the fundamental idea remains consistent.
Using Primitive Data Types in Variables
Variables act as containers for storing data values. We use primitive data types to declare the kind of data these variables will hold and how much memory they’ll use. Here’s how to effectively declare and initialize variables with primitive data types.
// Declaring an integer variable int score; // Initializing the variable score = 100; // Declaring and initializing in one line int livesRemaining = 3; // Working with floating-point numbers double heightInMeters = 1.85; float weightInKilos = 75.0f; // Using characters and booleans char grade = 'A'; boolean isGameOver = false;
Learning how to declare and initialize variables correctly is crucial, as it will set you up for success in constructing more elaborate structures down the road.
Arithmetic Operations with Primitive Data Types
Once you have variables, you’ll likely need to perform arithmetic operations. The following examples will guide you through some basic arithmetic using primitive data types.
// Adding two integers int sum = 10 + 15; // Subtracting two numbers double difference = 100.5 - 50.25; // Multiplying integers int product = 12 * 12; // Dividing numbers float divisionResult = 200.0f / 3.0f; // Combining variables and literals in an expression int x = 5; int y = 7; int result = x * y + 10;
Understanding these operations will open the door to manipulating data in your programs, allowing for dynamic and interactive coding experiences.
Logistical Operations with Booleans
Booleans aren’t just for keeping track of simple true or false states; they’re also vital in logical operations, such as comparisons and conditions. Let’s look at some examples of logical operations where booleans play a key role.
// Logical AND operation boolean isAdultAndVoter = (age >= 18) && (isCitizen == true); // Logical OR operation boolean canDrive = (hasLicense == true) || (hasPermit == true); // Logical NOT operation boolean isMinor = !(age >= 18); // Conditional assignment using booleans boolean isLeap = (year % 4 == 0) ? true : false;
These logical operations will be fundamental as you start to write code that needs to make decisions based on various conditions. Booleans provide the control you’ll need when branching into different paths of your program’s execution.As you delve deeper into programming, you’ll often encounter situations where you need to compare values or determine the sequence of operations. This is where relational and arithmetic operators come into play. Let’s explore how these operators interact with primitive data types through practical examples:
Comparing Values with Relational Operators
Relational operators allow you to compare two values and determine the relation between them. These comparisons are crucial for control structures like if-statements and loops. Here are some common relational operations:
// Checking for equality boolean isEqual = (10 == 10); // true // Inequality check boolean isNotEqual = (10 != 20); // true // Greater than boolean isGreaterThan = (15 > 10); // true // Less than boolean isLessThan = (5 = 5); // true // Less than or equal to boolean isLessOrEqual = (5 <= 5); // true
Sequence of Operations
Understanding the order in which operations occur is crucial for maintaining the integrity of your calculations. Computational tasks follow a sequence dictated by operator precedence, much like the order of operations in mathematics (PEMDAS/BODMAS). Here’s a quick example:
// Parentheses increase precedence int result = (2 + 3) * 4; // 20 // Multiplication is performed before addition without parentheses int resultWithoutParentheses = 2 + 3 * 4; // 14
Type Casting in Primitive Data Types
In the process of working with different types of primitives, you might need to convert from one type to another. This is known as type casting. There are two types of casting: implicit and explicit casting. Implicit casting happens without direct programmer intervention, while explicit casting requires it.
Here’s an example of implicit casting, where a smaller type is converted to a larger type automatically:
int myInt = 9; double myDouble = myInt; // Automatic casting: int to double
However, when assigning a larger type to a smaller type, you need to do explicit casting:
double myDouble = 9.78; int myInt = (int) myDouble; // Explicit casting: double to int
Be cautious with explicit casting; it can result in the loss of information, particularly with the truncation of decimal points when casting from floating-point types to integral types.
Constants with Primitive Data Types
There are times when you need to ensure that the value of a variable does not change throughout your program. You can achieve this by declaring the variable as a constant. Here’s an example of declaring constants in Java:
final int MAX_USERS = 100; final double PI = 3.14159;
Using constants is a best practice for values that remain static throughout your application, such as configuration parameters or mathematical constants like π (Pi).
Remember that the use of primitive data types and these operations is not limited to the examples provided. Your creativity and the needs of your program will guide you in harnessing these tools to their full potential. Keep practicing, and you’ll be wielding these fundamental concepts like a seasoned programmer in no time.When you’re comfortable with the basics of primitive data types, constants, and type casting, you can start exploring more intricate operations that involve these data types. This includes both arithmetic and bitwise operations, as well as string concatenation in languages like Java, which, while not a primitive data type, is a fundamental concept involving characters.
Bitwise Operations
Bitwise operators manipulate individual bits within an integer’s binary representation. These operations can be particularly useful in low-level programming, such as systems programming or when dealing with raw data processing. Here are some bitwise operation examples in Java:
// Assume int a = 60; binary: 0011 1100 // Assume int b = 13; binary: 0000 1101 // Bitwise AND int c = a & b; // binary: 0000 1100 // Bitwise OR c = a | b; // binary: 0011 1101 // Bitwise XOR c = a ^ b; // binary: 0011 0001 // Bitwise Complement c = ~a; // binary: 1100 0011 // Bitwise left shift (shifts left by 2 positions) c = a <> 2; // binary: 0000 1111
String Concatenation with Characters and Integers
In Java, strings are handled as objects, not primitive data types, but they often work closely with primitives. String concatenation is the process of appending one string to another, and you can also append primitives like integers and characters to a string:
String name = "Zenva"; int year = 2023; // Concatenate an integer with a string String greeting = "Welcome to " + name + " in the year " + year;
Increment and Decrement Operators
The increment and decrement operators are unary operators that increase or decrease the value of an integer by one. They’re used frequently in control structures, such as loops, to concise and effectively manage counter variables:
int i = 0; // Increment operator i++; // i becomes 1 // Decrement operator i--; // i becomes 0 again
These operators have both a prefix and postfix form, which can affect the order of operations:
// Prefix: Increment before using the value ++i; // Postfix: Use the value, then increment i++;
Using Arithmetic Operations for Combining Primitives
As you get more adept with primitives, you might find yourself using them in combination to perform more complex arithmetic:
int length = 10; int width = 5; // Calculating the area of a rectangle int area = length * width; // Finding the average of four exam scores int score1 = 85, score2 = 90, score3 = 88, score4 = 95; int average = (score1 + score2 + score3 + score4) / 4;
When you’re writing code involving arithmetic operations, remember to consider the data type of the result, especially when it comes to division, as you may need to cast to avoid losing precision if working with integers.
Understanding and putting these operations into practice will magnify your ability to solve problems effectively in programming. As you familiarize yourself with these examples, you’ll become increasingly confident in manipulating and utilizing primitive data types, which, in turn, will establish a solid foundation for diving into more advanced topics in software development.
Continue Your Learning Journey
Now that you have a foundational understanding of primitive data types, you might be wondering, “Where do I go from here?” The world of programming is vast, and there’s always more to learn and explore. At Zenva, we understand the importance of continuous education and practice in the tech field. That’s why we recommend diving into our Python Mini-Degree to broaden your programming skills. Python is a versatile and widely-used language that offers you the chance to grow in various arenas, from web development to data science.
Our Python Mini-Degree is designed to accommodate both beginners and those who already have a grasp of the basics. With our comprehensive courses, you can learn at your own pace and build practical projects to bolster your understanding and portfolio. Each step you take with us brings you closer to becoming a professional in the field.
For an even wider array of options, we also encourage you to explore our broader Programming courses. There, you’ll find a treasure trove of content covering different programming languages and disciplines that can pave the way for your burgeoning career in tech. Keep learning, keep coding, and turn your passion into a rewarding profession with Zenva.
Conclusion
In your journey through the world of programming, a solid grasp of primitive data types is not just an asset—it’s an essential tool in your developer’s kit. As you continue to grow and challenge yourself with new concepts and complex problems, you’ll find that these fundamentals will repeatedly serve as your guiding lighthouse. Whether you’re debugging intricate code or optimizing your next groundbreaking app, these are the principles that will keep you grounded and confident.
We invite you to further cement your knowledge and expand your horizons with Zenva. Our Python Mini-Degree awaits to guide you through the next steps, propelling you from foundational learning to real-world application. Embrace the journey ahead, strengthen your skills, and let’s code a future that’s limited only by your imagination. With Zenva, you’re never alone on the path to mastery.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
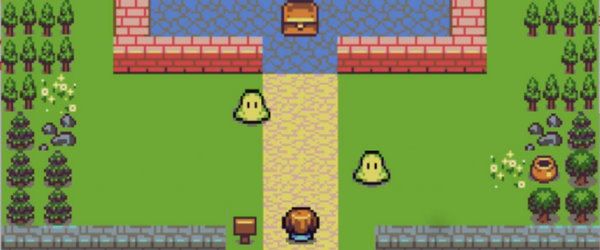
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.