Have you ever thought about the mechanics behind the games you play or the apps you use daily? Understanding these mechanics is key to not only appreciating technology but also creating your own. That’s where methods in programming come into play. Methods are fundamental building blocks in coding that enable programmers to create modular and reusable code. In this tutorial, we’ll explore methods inside out, providing you with knowledge that is both practical and applicable across various programming languages, with Python as our guiding example.
Whether you’re just starting your journey in programming or looking to solidify your understanding of methods, this tutorial aims to make the concept approachable and engaging. We’ll embark on this exploration together, unlocking the potentials of methods in games, applications, and beyond. So fasten your seatbelts—we’re about to dive into the world of methods, an adventure that’s essential for any budding or experienced coder’s toolkit!
Table of contents
What Are Methods in Programming?
Methods, sometimes referred to as functions or procedures in different programming languages, are like individual instructions or mini-programs within a larger program. They are designed to perform specific tasks and can be used multiple times throughout your code. This encourages not only a cleaner, more organized codebase but also an efficient way to handle repetitive tasks without rewriting the same code.
What Are Methods Used For?
In programming, methods are akin to tools in a toolbox—each with a different purpose and sometimes being versatile enough for multiple situations. Their uses range from performing calculations, handling user inputs, manipulating data, and controlling game behavior, to much more intricate operations.
Methods are the keystones in constructing modular code, which can be thought of as building your program with interlocking blocks rather than a mass of tangled strings.
Why Should I Learn About Methods?
Grasping the concept of methods is crucial for several reasons:
– They help you to write code that’s not only cleaner and easier to read but also easier to debug and maintain.
– Mastering methods empowers you to think abstractly about code, breaking down complex problems into manageable solutions.
– They are the stepping stones towards more advanced programming concepts like object-oriented programming.
– With methods, you can often enhance the performance of your program as you avoid the repetition of code.
Ultimately, methods are an essential part of programming literacy. By understanding them, you open the door to a world of efficient and sophisticated coding. Let’s continue to the next sections where we’ll see methods in action through Python code examples!
Defining and Calling Methods in Python
Before we can use a method, we have to define it. In Python, this is done using the ‘def’ keyword, followed by the name of the method and a pair of parentheses that may include parameters. Let’s see this in action:
def greet(): print("Hello, World!")
To call the method, you simply use its name followed by parentheses:
greet() # Output: Hello, World!
This simple mechanism underpins much of Python coding. With it, you can start creating blocks of code that can be called from anywhere in your program.
Adding Parameters to Methods
Parameters allow methods to process different data inputs and produce varied outputs. They are specified within the parentheses of the method definition. Here’s an example using parameters to personalize a greeting:
def greet(name): print("Hello, " + name + "!") greet("Alice") # Output: Hello, Alice! greet("Bob") # Output: Hello, Bob!
By incorporating parameters, your methods become more flexible and powerful, able to handle different scenarios smoothly.
Returning Values from Methods
Methods can also return values using the ‘return’ statement. This turns a method into a kind of ‘value-producing machine’. Here’s an example where we calculate the square of a number:
def square(number): return number * number result = square(4) print(result) # Output: 16
Once a method returns a value, you can use it as you would use any other value, like storing it in a variable or using it in expressions.
Using Multiple Parameters
Methods aren’t limited to a single parameter. You can define several parameters to handle multiple pieces of data:
def add_numbers(num1, num2): return num1 + num2 sum_result = add_numbers(10, 5) print(sum_result) # Output: 15
This concept is particularly handy when you’re looking to perform operations that require more than one input value, thereby broadening the method’s application.
Default Parameter Values
In Python, you can set default values for parameters, which will be used in case no argument is passed during a method call. This can save you time and make your method more user-friendly:
def greet(name="there"): print("Hello, " + name + "!") greet("Alice") # Output: Hello, Alice! greet() # Output: Hello, there!
With default parameters, your methods gain flexibility, allowing them to be called with fewer arguments when appropriate.
Keyword Arguments
Finally, let’s talk about keyword arguments. They allow you to pass arguments to methods in any order by specifying the name of the parameter:
def print_info(name, age): print("Name:", name, ", Age:", age) print_info(age=30, name="Alice") # Output: Name: Alice, Age: 30
This feature can be particularly useful in methods with a large number of parameters, as it enhances code readability by explicitly naming the purpose of each argument.Continuing our exploration of methods in Python, let’s delve into more complex scenarios that reflect real-world applications. We will work through several code examples that highlight different aspects of methods and how they can be used to streamline your programming.
Handling Variable Number of Arguments
There might be cases where you want a method to accept a varying number of arguments. Python allows for this through *args for positional arguments and **kwargs for keyword arguments.
def print_multiple(*args): for arg in args: print(arg) print_multiple('apple', 'banana', 'cherry') # Outputs apple, banana, cherry on new lines
Using **kwargs, you can handle named arguments that have not been previously defined:
def print_pet_names(**kwargs): for pet, name in kwargs.items(): print(f"{pet}: {name}") print_pet_names(dog="Rex", cat="Whiskers", fish="Bubbles") # Outputs dog: Rex, cat: Whiskers, fish: Bubbles on new lines
These techniques are incredibly useful when defining methods that need to be flexible with the number and type of arguments they receive.
Nesting Methods
Methods can be defined within other methods. This nested method approach can help in organizing code into a more readable and manageable structure.
def outer_method(text): def inner_method(): return text.upper() return inner_method() # The outer method calls the inner method print(outer_method('hello')) # Output: HELLO
This method-in-method structure is particularly beneficial when there’s a piece of functionality that’s only relevant within the scope of a larger operation.
Recursive Methods
Recurring methods are methods that call themselves. They are typically used to solve problems that can be broken down into smaller, identical problems, such as mathematical sequences.
def factorial(n): if n == 1: return 1 else: return n * factorial(n - 1) print(factorial(5)) # Output: 120
Recursion can be an elegant solution but also requires careful handling to avoid infinite loops and to ensure there’s always a base case to terminate the recursion.
Lambda Functions
Lambda functions are an example of anonymous methods in Python. They are defined by the lambda keyword and can be used where you need a method for a short period of time.
addition = lambda x, y: x + y print(addition(5, 7)) # Output: 12
Lambda functions are often used in higher-order functions, such as those that take other functions as arguments, like map, filter, and reduce.
Decorators
Decorators are a powerful feature in Python that allow you to modify the behavior of a method without changing its code. This is achieved by “wrapping” the method with another method.
def my_decorator(func): def wrapper(): print("Something is happening before the function is called.") func() print("Something is happening after the function is called.") return wrapper @my_decorator def say_hello(): print("Hello!") say_hello()
The output of this decorated method would include the additional prints from the wrapper. Decorators are a great example of how Python’s methods can be employed to add functionality in a clean, readable manner.
Method Documentation Strings
Documenting methods in Python is not only best practice, but also extremely helpful for someone else reading your code, or even you coming back to it after some time. Python uses documentation strings, or docstrings, which are denoted by triple quotes.
def multiply(num1, num2): """Multiply two numbers together. Args: num1 (int): The first number. num2 (int): The second number. Returns: int: The result of multiplying num1 by num2. """ return num1 * num2 help(multiply) # Displays the docstring
Employing docstrings can drastically enhance the understandability and usability of your code.
Methods are truly the heart of programming in Python, and as you dive deeper into their uses, you’ll discover yet more ways they can make your coding experience more effective and enjoyable. Whether it’s through creating cleaner, more organized code, or building complex functionalities with decorators and recursion, mastering methods is a quest that offers endless opportunities to simplify and strengthen your programming craft.Methods are versatile, and they don’t just stand alone—they often interact with each other to perform complex tasks. Let’s delve deeper into such interactions with more Python code examples, which will showcase how you can layer methods for more sophisticated coding.
Chaining Methods
You can chain method calls together to execute multiple operations in a single line. This can make your code more concise and can be particularly useful when the output of one method needs to be the input of another.
class StringManipulator: def __init__(self, string): self.string = string def reverse(self): self.string = self.string[::-1] return self def uppercase(self): self.string = self.string.upper() return self def output(self): print(self.string) # Chaining methods StringManipulator('hello').reverse().uppercase().output() # Output: OLLEH
This example uses a class, which is a collection of methods that reflects a higher level of structuring your code with methods.
Generator Methods
Python supports a special type of method called a generator method, which can be used to create iterators. Such methods use the yield statement to return a sequence of values over time.
def countdown(num): while num > 0: yield num num -= 1 for number in countdown(5): print(number) # Outputs: 5 4 3 2 1 on new lines
Generators are an efficient way to handle sequences of data without the need to store them in memory all at once, which can be crucial for handling large datasets.
Method Overloading
Method overloading is the ability to define the same method multiple times with different implementations based on the arguments. Python does not support method overloading by default, but you can mimic this behavior using default arguments or variable-length argument lists.
def product(a, b=1): return a * b print(product(5)) # Output: 5 print(product(5, 2)) # Output: 10
Here, the method ‘product’ can behave differently depending on the number of arguments passed to it, thus simulating overloading.
Error Handling in Methods
Robust code requires error handling to manage exceptions that occur during execution. You can add error handling in a method to gracefully deal with errors.
def divide(a, b): try: result = a / b except ZeroDivisionError: print("You can't divide by zero!") else: print(f"Result is {result}") divide(10, 2) # Output: Result is 5.0 divide(10, 0) # Output: You can't divide by zero!
In this example, the ‘divide’ method handles a potential division by zero, preventing the program from crashing.
Using Methods with Data Structures
Methods can also be useful for manipulating data within various data structures. For instance, you can define a method to process elements in a list.
def square_elements(numbers): return [number**2 for number in numbers] numbers = [1, 2, 3, 4, 5] squared_numbers = square_elements(numbers) print(squared_numbers) # Output: [1, 4, 9, 16, 25]
Methods like these can encapsulate logic that works with lists, dictionaries, sets, and other data structures, making your main code more readable.
Asynchronous Methods
Python also supports asynchronous methods, which are essential when dealing with IO-bound and high-latency operations. This allows other parts of your code to run while waiting for the operation to complete.
import asyncio async def fetch_data(delay): print("Start fetching") await asyncio.sleep(delay) print("Done fetching") return {'data': 1} async def print_numbers(): for i in range(10): print(i) await asyncio.sleep(0.25) async def main(): await asyncio.gather(fetch_data(2), print_numbers()) asyncio.run(main())
This example uses async methods to fetch data and print numbers concurrently, illustrating the power of asynchronous programming in Python.
Learning and mastering these additional facets of methods in Python will embellish your code with enhanced structure, clear data flow, and robust error handling, positioning you well on your path to becoming a proficient coder. Methods are a fundamental concept that translates across different programming languages, and the underlying principles you’ve seen here are widely applicable. Keep practicing, and you will find that methods open up a world of possibilities in your programming projects!
Where to Go Next in Your Python Journey
Embarking on the journey of mastering Python is an exhilarating experience, opening doors to numerous possibilities in the tech industry. If you’ve found a passion for programming methods or if the glimpse into Python’s capabilities has sparked your interest, consider taking the next step in your learning adventure. Our Python Mini-Degree presents a well-rounded curriculum that will guide you from the basics to the more complex aspects of Python programming. Through this course, you will gain hands-on experience by creating games, exploring algorithms, and developing real-world applications.
Furthermore, if you want to diversify your coding skills or explore other languages and technologies, our comprehensive collection of Programming Courses offers a wide array of content, from beginner to professional levels. With Zenva, the path from novice to professional is engaging, flexible, and designed to fit your personal pace and style. Every step you take with us is a step towards achieving your career goals and deepening your passion for technology. Keep learning, keep coding, and let’s build the future together.
Conclusion
As you can see, methods in Python are a gateway to building more efficient, maintainable, and scalable code. They form the backbone of not just Python, but virtually all programming languages. By mastering methods, you’re equipping yourself with a tool that will repeatedly prove its worth, whether you’re automating tasks, developing cutting-edge software, or creating captivating games. The road to programming mastery is an endless adventure, and understanding methods is a vital milestone on this journey.
To continue expanding your horizons, we invite you to join us at Zenva Academy, where learning is synonymous with doing. Our Python Mini-Degree is an actionable pathway to take your Python skills from foundational to extraordinary. Enroll now and be part of a thriving community of learners and professionals who share your passion for programming. Propel your career forward and write the code that shapes tomorrow.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
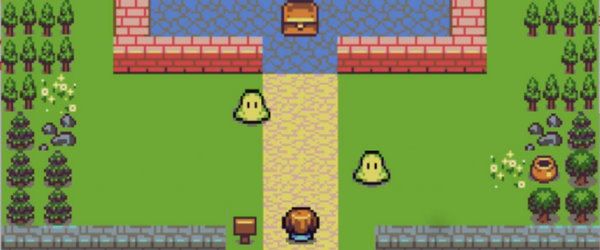
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.