Understanding and constructing graphical user interfaces (GUI) is a foundational skill in game development. One of the most important tools for creating GUIs within the Python SDK is Pygame GUI. In this enlightening tutorial, we will delve into this aspect of the Python SDK and efficiently hone your GUI creation skills.
Table of contents
What is Pygame GUI?
Pygame GUI is a module for Python, typically utilized alongside the Pygame library, to help with creating graphical interfaces in your game projects. Unlike more general-purpose tools, it’s tailored to meet the specific needs of game developers, with the ability to incorporate visually rich images, typography and interaction elements.
What is it for?
Pygame GUI is essential for creating user-friendly interfaces in an intuitive and straightforward manner. It aids developers in the construction of elements such as buttons, sliders, windows, and more. All these components contribute to an engaging and interactive user experience which plays a crucial role in the success of any game.
Why should I learn it?
Mastering Pygame GUI can significantly elevate the aesthetics and overall success of your gaming projects. By fostering players’ immersion and engagement through visually appealing and interactive menus, scoreboards or control panels, you create a more fulfilling gaming experience. Furthermore, Pygame GUI serves as one of the most learner-friendly tools for GUI creation, rendering it ideal for coders at the start of their journey and beyond.
Getting Started with Pygame GUI
To use Pygame GUI, you first need to import it into your project. This is done with the following line of code:
import pygame_gui
Note that you should already have the Pygame library installed and imported into your project before this operation.
Creating a User Interface Manager
A key component of using Pygame GUI is the User Interface Manager, which allows for easy management of interface elements. Here’s how you create it:
manager = pygame_gui.UIManager((800, 600))
We have just created a User Interface Manager for a window that’s 800 pixels wide and 600 pixels high.
Creating Interface Elements
With the manager in place, you can now start adding various user interface elements. Let’s start with a simple button:
button = pygame_gui.elements.UIButton(relative_rect=pygame.Rect((350, 275), (100, 50)),text='Play', manager=manager)
This code creates a button labeled ‘Play’ and positions it at the center of the window we defined earlier.
Creating other elements such as textboxes and labels follows a similar pattern, using different constructors from the pygame_gui.elements module. For instance, here’s how you create a basic label:
label = pygame_gui.elements.UILabel(relative_rect=pygame.Rect((400, 325), (100, 50)), text='Score: 0', manager=manager)
Updating and Drawing the GUI
Lastly, in your game loop, you should call the update and draw_ui methods on your manager to ensure that your GUI is properly rendered and updated:
time_delta = clock.tick(60)/1000.0 manager.update(time_delta) manager.draw_ui(window_surface)
With these steps, you’ve created a basic GUI in Pygame!
Customizing GUI Elements
Pygame GUI also gives you control over the aesthetics of your user interface elements. For instance, you may want to change the color of your button when it’s hovered over or clicked. This can be achieved by creating and using a UI theme:
theme = {'button': {'hovered_bg_color': '#FF0000', 'pressed_bg_color': '#00FF00'}} manager = pygame_gui.UIManager((800, 600), theme)
This sets the button’s background color to red when hovered over, and to green when pressed. No change is needed when creating the button itself, since these settings are automatically applied by the manager.
Handling GUI Events
You can also register and handle events relating to your UI elements. For instance, you might want to do something when your button is clicked:
for event in pygame.event.get(): if event.type == pygame.USEREVENT: if event.user_type == pygame_gui.UI_BUTTON_PRESSED: if event.ui_element == button: print("Button was clicked!")
This loop checks all events, and if a button was clicked, it prints a message. There are plenty of other events you can check for, and more in-depth handling can be done using event methods.
Interacting with Elements
Certain elements have their own methods for changing their state or value. The UILabel we created earlier, for example, can have its text changed with the following code:
label.set_text('Score: 100')
This will immediately change the label’s text to ‘Score: 100’. Other elements have their own unique methods, as dictated by their function and purpose.
Organizing Elements
When creating more complex GUIs, you might want to group related elements together. This can be done by creating a UI panel:
panel = pygame_gui.elements.UIPanel(pygame.Rect((50, 50), (700, 500)), manager=manager, anchors={'left': 'left', 'right': 'right', 'top': 'top', 'bottom': 'bottom'})
All elements that are created with this panel as their manager will be displayed inside the panel and move with it. In this way, you can create and manage complex UI layouts with relative ease.
With these tools at your disposal, you have the ability to create versatile and appealing user interfaces for your game projects. All you need is a little practice and creativity!
Creating Interactive Menus
Creating interactive menus in Pygame GUI is a breeze. Let’s start by creating a drop-down menu:
dropdown = pygame_gui.elements.UIDropDownMenu(['Option 1', 'Option 2', 'Option 3'], 'Option 1', pygame.Rect((100, 100), (200, 30)), manager)
This creates a drop-down menu with three options, the first one being initially selected.
Event Handling with UIDropDownMenu
Events can also be handled with the dropdown menus. To react to a selection change in our dropdown menu, we would use the following code:
if event.type == pygame.USEREVENT: if event.user_type == pygame_gui.UI_DROP_DOWN_MENU_CHANGED: if event.ui_element == dropdown: print(f'Selected option: {event.text}')
Every time a new option is selected, the event’s text property will hold the name of the new option. We can print this to the console to verify what has been chosen.
Text Entry
Allowing players to enter text can be crucial in certain games. This can simply be done by creating a UITextEntryLine:
entry = pygame_gui.elements.UITextEntryLine(pygame.Rect((100, 150), (200, 30)), manager)
Event Handling with UITextEntryLine
Event handling with text entry is similar to the previous examples. If we want to capture the text entered by the player, we can use the following code:
if event.type == pygame.USEREVENT: if event.user_type == pygame_gui.UI_TEXT_ENTRY_FINISHED: if event.ui_element == entry: print('Entered text: ', entry.get_text())
This will print the text entered by the player when they finish inputting the text by pressing Enter.
Toggle Switch
A toggle switch can be a clean and simple way for a player to choose between two options. Here’s how you can create one:
toggle_switch = pygame_gui.elements.UIToggleSwitch(pygame.Rect((100, 200), (100, 30)), 'OFF', 'On', manager)
Note that the strings ‘OFF’ and ‘On’ are the labels used by Pygame GUI on the toggle switch, with the first one being the default state. The `UIToggleSwitch` has a `toggle()` method, to change its state programmatically.
The Importance of Pygame GUI
Given its tremendous versatility, easy-to-use syntax and flexibility, Pygame GUI can be regarded as a vital tool in the repertoire of each budding game developer. We strongly believe that Pygame GUI is one of the best tools for GUI creation, especially for games, and we encourage learners to master its potential!
Where to Go Next
Having taken this significant stride in improving your Python and game development skills through learning Pygame GUI, it is an excellent time to think about your next steps. Continual learning and constant practice are vital to actualizing your full potential as a programmer and game developer.
Our Python Mini-Degree is a comprehensive collection of courses designed to further strengthen your Python programming. With a wide range of topics including coding basics, algorithms, object-oriented programming, game development, and app development, you’ll gain practical skills and create tangible projects. The Python Mini-Degree is a perfect opportunity for those who are looking to delve deeper into the versatile world of Python programming.
Looking for more specific learning content? Check out our full roster of Python courses. We offer content ranging from beginner to professional levels, featuring over 250 in-depth courses. Keep learning, coding, and creating with Zenva Academy!
Conclusion
We just delved into the fascinating aspects of Pygame GUI, a cornerstone tool in game development. With its intuitive syntax and flexible features, it’s a powerful stepping stone on your quest to create engaging and interactive game projects. The mastery of Pygame GUI not only adds a vital skill to your game development toolbox but also opens the doorway to a myriad of creative possibilities for user interface design.
Excited to wield your newfound knowledge and create your next milestone project? Further your learning journey with our comprehensive Python Mini-Degree. With Zenva, you’re on your way to becoming a confident, proficient, and innovative game developer. Let’s make learning to code easy and accessible together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
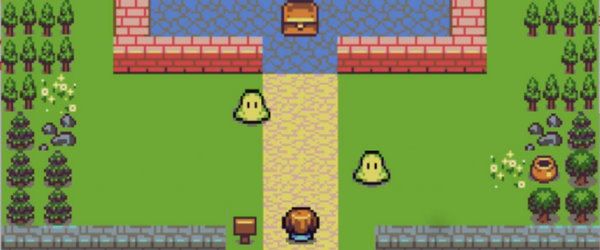
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.