Welcome to this comprehensive tutorial on the Pygame ‘blit’ function, a game-changing method that will enrich your understanding of game development in Python. With Pygame ‘blit’, creating dynamic and fascinating graphics becomes a breeze. So, whether you’re starting your coding journey or looking to expand your existing programming skills, this tutorial promises valuable knowledge and ultimate fun.
Table of contents
What is Pygame ‘blit’?
Before delving into examples and applications, it’s necessary to understand what ‘blit’ is. Pygame ‘blit’ is a function used in game development to draw surfaces onto a screen, making it essential for crafting visuals within your games.
So, why should I learn it?
Why is ‘blit’ so vital, you may wonder. Blit’ is the cornerstone of Pygame’s graphics. Knowing how to use ‘blit’ will enable you to implement vibrant graphics into your games, making them enjoyable, engaging and visually appealing. It’s a method that can breathe life into your game designs.
What is it for?
The main purpose of the ‘blit’ function? It transfers pixels from one image to another. This is the mechanism behind moving characters, changing backgrounds and much of the dynamism found in games. Mastering ‘blit’ opens up unlimited game design possibilities, enrichening your game development journey.
Starting with Pygame ‘blit’
To begin our exploration of Pygame ‘blit’, we first need to initialize Pygame and create a game display:
import pygame pygame.init() # Setting Display game_display = pygame.display.set_mode((800,600))
In the game display mode, we can specify the width and height of the game window. This will create a display surface that we can draw on with other Pygame functions.
Basic Usage of ‘blit’
We next create an image surface in Pygame, which we can place onto our game display using ‘blit. In this next example, we will create a red square and ‘blit’ it to the center of our game window:
# Creating Surface square = pygame.Surface((50,50)) square.fill((255,0,0)) # Red color # Blitting Surface game_display.blit(square, (375,275)) # Positioning at the center
Notice that our ‘blit’ function takes two arguments – the surface we are transferring (our red square), and the coordinates we want it placed on (the center of our game display).
Manipulating ‘blit’ for Complex Surfaces
‘Blit’ shines in moving complex images around the screen. For instance, if you have a sprite for a game character, you can move it using ‘blit’:
# Loading character sprite character = pygame.image.load('character.png') # Blitting character at position (x,y) x = 100 y = 100 game_display.blit(character, (x,y))
This allows our static character to begin moving around our game window. Now let’s make our character move. Change the position where we want to ‘blit’ our character:
# Updating character position x += 5 y += 2 # Blitting at new position game_display.blit(character, (x,y))
By doing this, the character will appear to move as you keep updating the game display.
Continued Usage and Abstract Application of ‘blit’
Moving images around the screen is a key aspect of game development, but the use of ‘blit’ extends to more complex situations too. When it comes to manipulating surfaces in Pygame, ‘blit’ is our go-to function.
Consider having a series of images, say frames of a character animation. You could sequence these frames using a loop and ‘blit’ to create an animation:
# Assuming we have list of frame images frames = [pygame.image.load(f'frame{i}.png') for i in range(6)] # Looping through frames x, y = 100, 100 for frame in frames: game_display.blit(frame, (x,y)) pygame.display.update()
Here, ‘blit’ enables us to sequentially display the frames of our animation, making the character appear to move or perform an action.
‘Blit’ can be used in conjunction with a variety of Pygame functionalities. For instance, you can use ‘blit’ to display text:
# Creating font and rendering text font = pygame.font.Font(None, 50) text = font.render("Hello, Pygame!", True, (255,255,255)) # Blitting text text_position = (200, 200) game_display.blit(text, text_position)
Here, ‘blit’ takes the rendered text and “blits” it onto our game display surface, enabling us to display dynamic text within our game window.
Another cool application of ‘blit’ is to implement parallax scrolling backgrounds, which adds an illusion of depth to 2D games.
# Assuming we have a larger background image bg_image = pygame.image.load('background.png') # Initial blit x = 0 game_display.blit(bg_image, (x,0)) # Next position blit for scrolling effect x -= 1 game_display.blit(bg_image, (x,0))
Here, by continuously decrementing the x value, you create a feeling of movement, as if the character is traversing through the game world.
In summary, the ‘blit’ function in Pygame is not just a tool, but a Swiss army knife for Python game development. It’s essential for drawing any sort of surface onto your game display, be it static or dynamic images, animated sequences, text, or even parallax scrolling backgrounds.
Advanced Applications of ‘blit’
As you continue to dive deeper into game development, you’ll see that the Pygame ‘blit’ function offers even more versatility and control over positioning graphics. Let’s explore a few advanced use cases of this function.
Rotating objects
You can rotate your player or any surface in a game. For instance, let’s take an example of rotating a spaceship:
# Assuming spaceship image is loaded in 'spaceship' angle = 0 while True: # ... game event handling... # Rotating and blitting spaceship rotated_spaceship = pygame.transform.rotate(spaceship, angle) game_display.blit(rotated_spaceship, (200,200)) # Increasing angle for next rotation angle += 1
With each game loop, the spaceship will rotate by 1 degree, giving a spinning animation effect.
Scaled blitting
The scale and size of sprites and surfaces is another crucial aspect of game aesthetics. ‘blit’ function can work seamlessly with pygame.transform.scale() to adjust the dimensions. Suppose we want to double the size of our spaceship surface:
# Getting current size width, height = spaceship.get_size() # Scaling spaceship = pygame.transform.scale(spaceship, (width*2, height*2)) # Now we blit our scaled spaceship game_display.blit(spaceship, (200,200))
Scaling adds another dimension of interactivity and dynamics to your Pygame projects.
Blitting with transparency
Transparent surfaces, or ‘alpha surfaces’, add to the layering effect in games, especially for creating overlays, shadows, and highlighting effects. ‘blit()’ function supports blitting alpha surfaces:
# Creating a semi-transparent surface overlay = pygame.Surface((100,100)).convert_alpha() overlay.fill((255,0,0,128)) # The last value represents alpha (128 semi-transparent) # Blitting the overlay game_display.blit(overlay, (200,200))
You’ll see a semi-transparent red square at x=200, y=200. Full transparency would make the surface invisible, while a lower alpha value will make the surface more opaque.
Flipping sprites and images
Sometimes, for using single sprite for left-right or up-down movements, flipping can be handy:
# Assuming 'character' image is loaded flipped_character = pygame.transform.flip(character, True, False) # Now we blit our flipped character game_display.blit(flipped_character, (200,200))
Here, the character image is horizontally flipped. If you move your character towards left, this sprite could be blitted to give the sense of leftward movement.
As we’ve seen, ‘blit’ function is powerful, versatile and an integral part of Pygame’s graphics rendering. It provides endless possibilities – from simple sprite movements to animations, scrolling backgrounds, dynamic scaling, rotation, transparency and so much more. So get exploring and have fun creating with Pygame and ‘blit’!
Embrace your coding journey and step into the realm of infinite creativity and opportunities with Zenva’s Python Mini-Degree. This comprehensive learning path dives into various aspects of Python programming such as coding fundamentals, game and app development, algorithms, and much more. It’s an ideal destination for beginners and experienced programmers alike, providing the opportunity to learn at your own pace and earn certificates at the end.
From building your own games and apps to crafting AI chatbots, you’ll gain hands-on experience via practical projects, quizzes, and coding challenges. And what’s more, our content is regularly refreshed to keep you up-to-date with the latest industry trends and developments, making this an evergreen learning resource for all Python enthusiasts.
If you’re looking for a wider variety of Python courses, we invite you to check out our extensive collection of Python courses. Always remember, we at Zenva are committed to transforming you from being a beginner to a professional. Keep learning, keep growing.
Conclusion
Learning to use the Pygame ‘blit’ function propels your game development journey to impressive new heights. It sets a robust foundation for creating fascinating visuals, lively animations, and dynamic gaming experiences. With the power of ‘blit’, you can transform fun project ideas into live, engaging Python games.
Enhance your skill set and take the Python game development leap with us at Zenva. Our Python Mini-Degree offers thorough and thoughtful modules that set you on the right track to becoming a proficient Python developer. Happy coding, and always remember – the sky’s the limit when programming with Pygame and Python.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
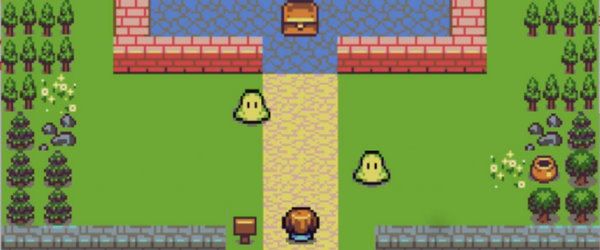
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.