Commenting code is important for readability – which makes JavaScript DocStrings an important thing to learn!
JavaScript remains one of the most popular and dynamic languages used in the web development world, with its application noticeable in the client-side and server-side web development. As its use becomes more widespread, there is an increasing need for a tool to make codes more understandable – enter JavaScript Docstrings. Docstrings not only aid in the process of coding, but they enhance the readability and maintainability of your codebase. Ready to find out what they’re all about? Let’s get started.
Table of contents
What are JavaScript Docstrings?
Firstly, it’s key to understand what we’re talking about. Docstrings, or Documentation Strings, are essentially descriptive text strings that explain what a segment of code or a function does in your JavaScript code. They give insight into the purpose and functionality of a particular code snippet.
Why Are Docstrings Useful?
Now you know what docstrings are, you might be wondering “why should I learn this?” The answer is simple: docstrings drastically improve your code’s readability.
- They make it easier for other developers (or even your future self) to understand your code logic.
- They save time, as comprehensive docstrings prevent developers having to read through an entire script just to understand specific aspects.
- They aid in debugging as the crux of the function is summarized.
The usage of JS docstrings stands as a best practice in coding documentation, a must-know for aspiring and accomplished developers.
How to Write a Docstring in JavaScript
Let’s get straight into the practical application. Docstrings in JavaScript can be added with the help of multiline comments, starting and ending with /\*\* and \*/ respectively. Below the docstring, you define your function.
/** * This is a JavaScript docstring * Here you explain the purpose of the following function */ function myFunction() { // your code here }
Describe Parameters With @param
Are your functions using parameters? Inside docstrings, you can list and describe these parameters using the @param JSDoc tag. Here’s an example:
/** * This function adds two numbers * @param {number} a - The first number * @param {number} b - The second number */ function addNumbers(a, b) { return a + b; }
Defining Return Values With @returns
In case your function returns a value, you can highlight this using the @returns (or @return) JSDoc tag.
/** * This function subtracts two numbers * @param {number} a - The first number * @param {number} b - The second number * @returns {number} The difference between the two numbers */ function subtractNumbers(a, b) { return a - b; }
Reading a JavaScript Docstring
Finally, it’s important to know how to interpret the docstrings as a reader. Let’s look at an example docstring:
/** * This function divides two numbers * @param {number} a - The numerator * @param {number} b - The denominator * @returns {number} The division of the numerator by the denominator * @throws {DivideByZeroException} When attempt is made to divide by zero */ function divideNumbers(a, b){ if(b == 0) throw "DivideByZeroException"; return a/b; }
In the above example:
- The docstring describes the function as performing a division operation.
- The @param tags describe the two parameters being parsed in the function – the numerator (a) and the denominator (b).
- The @returns tag shows that the function returns the division of a by b.
- The @throws keyword shows that an exception will be thrown if an attempt is made to divide by zero.
This helps any user of the function to quickly grasp what the function does, its inputs, output, and possible exception.
Defining Examples With @example
You can guide developers using your function by adding an example of how to use the function with the @example JSDoc tag.
/** * This function multiplies two numbers * @param {number} a - The first number * @param {number} b - The second number * @returns {number} The multiplication of the two numbers * @example * // Returns 6 * multiplyNumbers(2, 3); */ function multiplyNumbers(a, b) { return a * b; }
Defining Optional Parameters With [paramName]
It’s not always required for a function to have all its parameters. You can indicate optional parameters within Javascript Docstrings. Simply enclose the parameter name within brackets [] in the @param tag.
/** * A greeting function * @param {string} name - The name to greet * @param {string} [timeOfDay=] - The time of day * @example * // Returns "Good morning, Sam" * greeting("Sam","morning"); * // Returns "Hello, Sam" * greeting("Sam"); */ function greeting(name, timeOfDay){ if(timeOfDay) return `Good ${timeOfDay}, ${name}`; return `Hello, ${name}`; }
Describing a Default Parameter Value with @default
If a default value is already set for a parameter in a function, you can indicate this in the JavaScript docstring with the @default tag.
/** * Enumeration for tri-state values. * @readonly * @enum {number} * @default */ var STATE = { ON: 1, OFF: 0, UNKNOWN: -1 };
Encapsulating Tags With @function and @var
The @function and @var tags can aid readability in your Docstring by enabling you to encapsulate your Docstring.
/** @function */ var square = function(n) { return n*n; }; /** @var {number} */ var foo = 42;
JavaScript Docstrings are undeniably a potent tool in your JavaScript toolkit. Do well to include them in your coding process for an enhanced and simplified coding experience.
How to Keep Learning and Where to Go Next
The world of web development is vast and expanding every day. To stay ahead of the curve, continuous learning and application of new knowledge are key.
At Zenva, we provide a wide array of courses for beginners, intermediate learners, and professionals. Our courses in programming, game development, and artificial intelligence have been meticulously designed to boost your career and empower you with the technical skills needed in today’s digital era.
For those keen on diving deeper into web development, we recommend our comprehensive Full-Stack Web Development Mini-Degree.
The Full-Stack Web Development Mini-Degree covers everything you need to become an industry-ready full-stack web developer. During the course, you’ll learn:
- HTML, CSS, and JavaScript – the foundational components of web development
- Front-end and back-end development
- Building server-side apps and REST APIs
- Version control techniques
- Deployment methods with AWS and Azure.
All our courses, including the Full-Stack Web Development Mini-Degree, are designed to accommodate learners from all backgrounds and skill levels. Through project-based learning and interactive lessons, you’ll have the chance to apply what you’ve learned in a practical setting.
By the end of this program, you will have developed a professional portfolio showcasing your full-stack web development skills. Beyond that, you will be part of a community of learners and developers where engagement fosters growth.
For those who are already past the basics and looking to reinforce their JavaScript skills, we offer specialized JavaScript courses.
At Zenva, we believe in bridging the gap between learning and doing. With modern and regularly updated content, we ensure you always stay up-to-date with the latest industry practices. Let’s take the next step in your learning journey, together.
Conclusion
JavaScript Docstrings can truly revolutionize your coding process. With these, your code becomes self-explanatory, more maintainable and more accessible to both you and other developers. Plus, they can help to create robust documentation for your software project with minimal effort.
Ready to take your learning further and embrace more industry-leading practices? Check out our comprehensive Full-Stack Web Development Mini-Degree. The leap to becoming a seasoned developer is just a course away. Join us at Zenva to start your journey today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
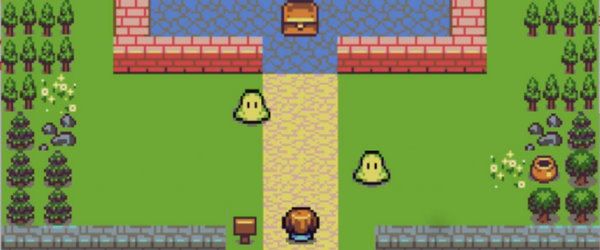
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.