Welcome to this exciting tutorial where we dive deep into the fascinating world of GDScript math. We aim to unveil the power and flexibility GDScript offers when it comes to evaluating and manipulating mathematical expressions. These skills will not only enrich your toolbox as a game developer but will also make creating complex gameplay mechanics a breeze.
Table of contents
What is GDScript Math?
GDScript is the scripting language used by the Godot game engine. Its math component, colloquially referred to as GDScript Math, is a collection of functions and formulas that allow you to perform mathematical operations. A profound understanding of GDScript Math could mean the difference between creating a mundanely flat game or an immersive gaming experience replete with intricate gameplay mechanics.
Why Should You Learn It?
Just as how the right stroke of a brush could bring a painting to life, the appropriate use of math could breathe life into your game. Even the simplest of games, from 2D platformers to puzzle games, rely heavily on math. Implementing physics for movement, creating procedural content, designing AI behaviours, all require a sound understanding of mathematical principles.
Learning GDScript Math will:
- Open doors to creating innovative gameplay mechanics.
- Equip you to handle and manipulate in-game physics.
- Enable you to construct complex AI behaviours.
Enhanced with real-life examples and self-contained code snippets, we’ve designed this tutorial to be accessible for beginners yet informative enough for experienced coders. Enjoy the journey as we explore the fascinating world of GDScript Math.
Basic Mathematical Operations
First, let’s familiarize ourselves with how GDScript handles basic mathematical operations such as addition, subtraction, multiplication, and division. These operations form the building blocks of any mathematical functionality.
GDScript follows the order of operations in mathematics, generally referred to by the acronym PEMDAS (Parentheses, Exponents, Multiplication and Division, Addition and Subtraction).
var a = 10 var b = 5 var addition = a + b # 15 var subtraction = a - b # 5 var multiplication = a * b # 50 var division = a / b # 2
Using Math Functions
GDScript includes a library of powerful math functions that can significantly simplify complex calculations.
For instance, the abs() function returns the absolute value of a number.
var c = -10 var abs_value = abs(c) # 10
The GDScript math library also includes functions that go beyond basic operations. trigonometric functions such as sin(), cos(), and tan():
var d = 1.57 # close approximation to pi/2 var sine = sin(d) # 1 var cosine = cos(d) # close to 0 var tangent = tan(d) # very large number
Random Numbers
GDScript gives developers the ability to generate random numbers, which is invaluable in creating dynamic and varied gaming experiences.
var random_num = randi() % 10 # a random integer between 0-9
You can also generate random floating point numbers using randf():
var random_float = randf() # a random float between 0 and 1
Remember to initialize the random number generator using randomize(). If not initialized, the sequence of random numbers will remain the same across different runs.
randomize() var random_num = randi() % 10
Math Constants
GDScript Math includes a set of mathematical constants that come in handy when performing complex calculations.
var pi_value = PI # 3.141592653589793238 var e_value = INF # Float Infinity (+inf) var nan_value = NAN # Not a Number (NaN)
Using these constants, various complex mathematical concepts can be represented within the GDScript in a very approachable and accommodative manner. You’ve seen a taste of the magic of math in GDScript, stick with us as we delve deeper.
Vector Operations
Vectors form the core of many gaming concepts, such as movement, force, and direction. GDScript provides a comprehensive set of operations and functions for dealing with vectors.
For creating 2D and 3D vectors, GDScript provides Vector2() and Vector3() functions respectively.
var vec_2D = Vector2(1,2) # a 2D vector (1,2) var vec_3D = Vector3(1,2,3) # a 3D vector (1,2,3)
Similar to basic operations, you can perform addition, subtraction, and multiplication operations on vectors.
var vec1 = Vector2(1,2) var vec2 = Vector2(3,4) var vec_add = vec1 + vec2 # Vector2(4,6) var vec_sub = vec1 - vec2 # Vector2(-2,-2) var vec_mul = vec1 * vec2 # Vector2(3,8)
Functions for Vector Operations
In addition to basic operations, GDScript provides a set of functions tailored towards vector manipulation.
You can calculate the dot product of two vectors using the dot() function:
var dot_product = vec1.dot(vec2) # 11
Similarly, the cross product of two 3-dimensional vectors can be calculated using cross() function:
var vec3 = Vector3(1,2,3) var vec4 = Vector3(4,5,6) var cross_product = vec3.cross(vec4) # Vector3(-3,6,-3)
The length() function returns the magnitude of the vector:
var vec_length = vec1.length() # 2.236
These vector operations and functions in GDScript hold the key to creating scenes with realistic movements, precise inter-object interactions, and accurate AI behaviours.
Interpolation and Easing
Interpolation and easing functions play a crucial role in creating smooth movements and transitions in gaming. They determine how the intermediate values between the two extremes are calculated and visualized on the screen.
The lerp() function provides linear interpolation:
# lerp(start, end, weight) var lerp_value = lerp(1, 10, 0.5) # outputs 5.5
For more complex easing effects, GDScript provides various easing functions like ease():
# ease(value, curve_type) # curve_type ranges from -1 (ease in) to 1 (ease out) var ease_value = ease(0.5, 1) # outputs 1
Acquiring these crucial skills in GDScript Math will not only help you create more engaging gameplay mechanics but will also make the development process more fun and effortless.
Complex Math Functions
Let’s now dive into some of the more complex GDScript math functions. These concepts pave the way for even more advanced game mechanics and bring a considerable depth to the development process.
Trigonometry
Trigonometry is fundamental to many aspects of game development, including rotations, circular motions, and waves. GDScript offers a set of robust trigonometric functions.
The atan2(y, x) function gives the angle between the positive X-axis and the point (x, y):
var angle = atan2(1,1) # 0.785
The deg2rad(degree) function converts degrees to radians, whereas rad2deg(rad) does the opposite:
var radians = deg2rad(30) # 0.524 rad var degrees = rad2deg(1) # 57.295 deg
Exponential and Logarithmic Functions
Exponential and logarithmic functions are necessary when you want to deal with values that increase or decrease exponentially. The implementation of growth rates, decay, or distance-based fall-off effects will require the use of these functions.
The pow(base, exponent) function raises a base to a certain exponent:
var power = pow(2,3) # 8
The log(x) and exp(x) functions return the natural logarithm and exponent of ‘x’, respectively:
var logarithm = log(10) # 2.302 var exponential = exp(1) # 2.718
Rounding Functions
Rounding numbers is yet another common requirement in game development. Whether for score calculation, timing or in-game currency, rounding functions are indispensable.
The round() function rounds a floating-point number to the nearest integer:
var rounded = round(2.6) # 3
You can also round up or down to the nearest integer with ceil() and floor():
var up = ceil(2.3) # 3 var down = floor(2.6) # 2
Modulo Operator
The modulo operator, represented by ‘%’, is used to obtain the remainder of a division operation. It’s extremely helpful when creating cyclic or repeating behaviors in your games.
var mod = 10 % 3 # 1
By harnessing the power of GDScript’s math functions, you can bring a high level of sophistication and polish to your games. We encourage you to experiment with these functions and see the magic unfold.
What’s Next?
As you continue your journey in GDScript and game design, remember that practical application holds the key. The more you use and experiment with these functions, the better you’ll understand how they can enhance your games. Don’t be afraid to explore the more complex concepts and dive into more intricate projects.
We invite you to check out our Godot Game Development Mini-Degree. This comprehensive offering deep-dives into cross-platform game building using the Godot 4 engine. From utilizing 2D and 3D assets and mastering GDScript to player-enemy combat and building sophisticated UI systems, this Mini-Degree has it all. Designed with project-based lessons and flexible learning options, it offers a wonderful opportunity to acquire the skills essential for a career in game development.
Another great way to continue expanding your game development skills is by delving into our broad collection of Godot courses. With Zenva, you can go from beginner to professional at your own pace. Keep coding, creating games, and most importantly, enjoy your learning journey.
Conclusion
GDScript Math is a potent tool that can greatly enhance the dynamics of your game development process. It expands your creative horizon, empowers you to breathe life into your virtual reality, and presents unlimited possibilities for creating diverse gameplay experiences.
Dive into our Godot Game Development Mini-Degree and continue your journey of creating engaging, dynamic, and immersive games. With GDScript Math as your beacon, unlock new dimensions in game development, and let your creations shine. Remember, the only limit is your imagination. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
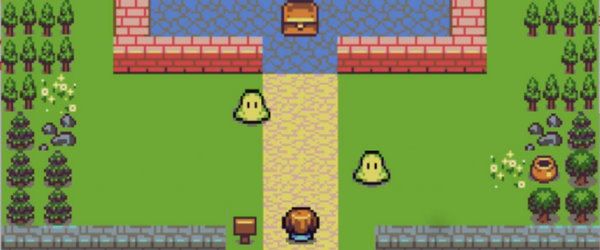
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.