Welcome, coding enthusiasts, to this comprehensive guide on GDScript for loops. Whether you’re a newcomer to coding or have programming experience, this article will deepen your understanding of for loops in GDScript, an essential aspect of scripting with the game engine Godot.
Table of contents
What is a GDScript For Loop?
A for loop in GDScript is similar to those in other programming languages. It’s a control flow statement, allowing us to iterate over a sequence such as a list, array, or range of numbers.
What is it For?
For loops are incredibly versatile in game development and coding in general. They can be used for a variety of tasks like running a chunk of code a specific number of times, traversing through arrays or lists, or implementing certain game mechanics.
Why Should You Learn it?
Understanding how the GDScript for loop operates is crucial for anyone interested in game development with the Godot engine. Mastering for loops can aid in writing efficient and effective code, making your game run smoothly and opening up a world of complex game mechanics.
Basic Implementation of GDScript For Loop
Let’s get started with a simple for loop in GDScript, iterating over a defined sequence of numbers.
for i in range(4): print(i)
The output will be:
0 1 2 3
This is a basic for loop that runs over a range of 0-3 (as the range function doesn’t include the end number), printing out each number in turn.
GDScript For Loop with Array
Now, let’s look at how we can use a for loop with an array in GDScript. In the following example, we are iterating over an array of strings.
var fruits = ["Apple", "Banana", "Cherry"] for fruit in fruits: print(fruit)
The output will be:
Apple Banana Cherry
In this example, the for loop iterates over each element of the array, and the print statement outputs each fruit one by one.
GDScript For Loop with Break and Continue
For loops can be controlled using break and continue statements. `Break` halts the loop prematurely, while `continue` skips to the next iteration. Here’s how you can use them in GDScript:
for i in range(8): if i == 4: break print(i)
This will output:
0 1 2 3
After hitting the number 4, the `break` statement stops the for loop. Note that 4 is not printed because the loop was halted before the print statement could execute.
Now, let’s see an example of using `continue`:
for i in range(6): if i == 2: continue print(i)
This will output:
0 1 3 4 5
Here, when the loop hits the number 2, the `continue` statement is triggered, which skips over the rest of the current iteration, thus preventing 2 from being printed.
GDScript For Loop with Enumerations
Enumerations can also be used with for loops in GDScript. An enumeration is a custom data type consisting of integral constants. Let’s write a for loop with an enumeration:
enum Week {Mon, Tue, Wed, Thu, Fri, Sat, Sun} for day in Week.values(): print(day)
This will output:
0 1 2 3 4 5 6
In this example, the loop iterates over each day of the week in the enumeration, printing the integer value associated with each day.
GDScript For Loop for Dictionaries
GDScript’s for loops are equally effective when working with dictionaries. A dictionary is a collection of key-value pairs, and you can use a for loop to iterate over these pairs. Here’s an example:
var person = {"name": "John", "age": 30, "city": "New York"} for key in person.keys(): print(key + ": " + str(person[key]))
The output will be:
name: John age: 30 city: New York
In this case, the loop iterates over each key in the dictionary and prints out both the key and its associated value.
GDScript For Loop for 2D Arrays
You can use for loops in GDScript to traverse 2D arrays as well. Here’s how:
var matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] for row in matrix: for num in row: print(num)
The output will be:
1 2 3 4 5 6 7 8 9
We make use of nested for loops to iterate through each row and then each number in that row, printing all the numbers in the matrix.
Nested GDScript For Loop
Nested for loops are also possible in GDScript. In a nested for loop, you have one loop inside another. It’s often used to work with multi-dimensional data structures. Here’s an example:
for i in range(3): for j in range(3): print("i: " + str(i) + ", j: " + str(j))
The output will be:
i: 0, j: 0 i: 0, j: 1 i: 0, j: 2 i: 1, j: 0 i: 1, j: 1 i: 1, j: 2 i: 2, j: 0 i: 2, j: 1 i: 2, j: 2
In this example, for every value of ‘i’, the inner loop runs three times, resulting in nine iterations in total.
Use of GDScript For Loops in Godot Engine
Practically speaking, in game development using the Godot Engine, you can leverage GDScript’s for loop in various cases. One such use-case is iterating over nodes:
for child_node in get_children(): child_node.hide()
Assuming this script is attached to a parent node, this snippet would iterate over all of the parent node’s children, hiding them in the process.
As you can see, GDScript for loops offer versatility and control, making them a vital tool in game development with Godot. Happy coding!
GDScript For Loop with Step Argument
GDScript also allows for a step argument in for loops, useful when you want the loop to iterate over a range with an increment other than one. Here’s how you can implement it:
for i in range(0, 10, 2): print(i)
The output will be:
0 2 4 6 8
In this example, the loop increments by 2 during each iteration, only printing even numbers from 0 to 8.
GDScript For Loop with Two Variables
GDScript for loops can also be used with two variables when iterating over a dictionary. Here’s an example:
var colors = {"red": "#FF0000", "green": "#00FF00", "blue": "#0000FF"} for key, value in colors: print("The hex code for " + key + " is " + value)
The output will show the name of each color with its respective hex color code.
Traversing Array Backwards with GDScript For Loop
Traversing arrays backwards is no different than forward, all you need is to adjust the ranges. Here is an example:
var numbers = [1, 2, 3, 4, 5] for i in range(numbers.size() - 1, -1, -1): print(numbers[i])
The output will be:
5 4 3 2 1
This for loop starts from the last index of the array and continues to the first index, with each iteration decrementing the index by 1, thus printing the array elements in reverse order.
GDScript For Loop in Practical Game Development
Besides these general programming usages, GDScript for loop also has some specific game development purposes. Let’s consider some use cases:
Suppose we want to apply gravity on all instances of a given type, we can write:
for body in get_tree().get_nodes_in_group("PhysicsBodies"): body.add_force(Vector2(0, 9.8 * body.mass), Vector2.ZERO)
In this example, a force simulating gravity is applied to all nodes in the PhysicsBodies group.
Another common use-case could be checking all nodes in a scene against a condition. For instance, if you want to check if all enemies have been defeated, you can do:
var enemies_defeated = true for enemy in get_tree().get_nodes_in_group("Enemies"): if enemy.alive: enemies_defeated = false break
This snippet will change the variable `enemies_defeated` to false as soon as it finds an enemy that is still alive, and exits the loop immediately.
You can see that the flexibility and power of GDScript’s for loop makes it a tool you will be frequently using during your journey into Godot game development.
Where to Go Next? How to Keep Learning?
Undoubtedly, the journey of learning Godot scripting is both rewarding and challenging, and mastering GDScript for loops is just the tip of the iceberg. The more you delve into it, the more exciting it becomes!
At Zenva, we aim to fuel that excitement through our courses that encompass a wide array of topics ranging from the basics to advanced game development and AI. Whether you are just getting started or are seeking to push your skills to the next level, our expansive catalog of over 250 supported courses has something for everyone.
The next power move on your game development journey could be our Godot Game Development Mini-Degree. This comprehensive collection of courses guides you in building cross-platform games using the powerful, open-source Godot engine. Learn to create assets, implement a variety of game mechanics, control gameplay flow, and so much more. As you progress through the degree, you’ll even build your own games, developing a strong portfolio to embark on your gaming career.
The curriculum is designed to be flexible and accessible, with courses led by experienced game developers, featuring live coding lessons, quizzes, and interactive lessons to ensure an immersive and practical learning experience.
If you’d like to explore more Godot-focused opportunities, you can also check out our broader collection of Godot Courses.
Remember, learning is a journey, not a destination. With every new function, command, or script mastered, you’re one step closer to creating incredible games with Godot. A universe of coding adventures awaits you at Zenva. So why wait? Get started with your coding journey today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
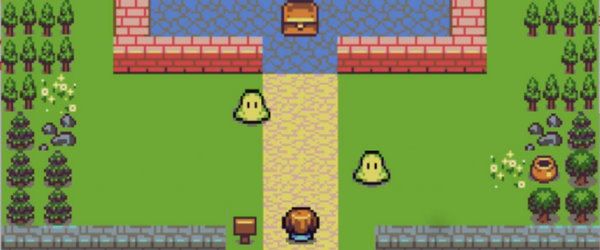
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.