Welcome to a comprehensive tutorial on GDScript export. As you journey through this piece, we will cover the ins and outs of this powerful feature inherent in the GDScript programming language, often utilized in Godot Engine. Regardless of your coding experience, this guide is curated to be as engaging as possible, illustrating features with digestible examples and explanations.
Table of contents
What is GDScript Export?
GDScript export is a keyword in the GDScript language which helps to expose variables in the editor. This handy trick allows for easier debugging and testing, saving significant time and effort.
What is GDScript Export Used For?
The export keyword in GDScript is predominantly used for manipulating variables directly in the Godot interface. It earns its value in its capacity to streamline both development and debugging processes.
Why Should You Learn About GDScript Export?
Mastering GDScript export can truly elevate your game development workflow. The power to change variables on the fly in the editor, without needing to dive into your script each time, can expedite the debugging process and make iterations quicker.
Skills in GDScript export are particularly prudent for aspiring developers using the Godot Engine, providing a real-world, applicable asset readily implementable in their projects. An understanding of GDScript export ensures a smoother coding journey, enabling developers to tackle more complex projects with confidence.
Basic Usage of GDScript Export
To start with, let’s have a look at how we can use the export keyword to expose a variable in the editor. For this, we’ll declare a variable and then use the export keyword before its declaration.
export var name = "Zenva"
In the above code snippet, we’ve created a string variable “name” and assigned it a default value “Zenva”. The export keyword before the variable declaration makes the variable visible in the editor, allowing us to change its contents directly from there.
Specifying a Type with Export
In GDScript, we can specify the type of an exported variable. This limits the types of values that can be assigned to this variable. Here is a code snippet that exports a variable of type int.
export(int) var number = 5
Now, if you attempt to assign a value that is not an int to the variable “number” in the editor, it will throw an error.
Exporting Arrays
Not only basic data types, but we can also export arrays in GDScript. Below is an example of exporting an array of integers:
export(Array, int) var numbers_array = [1,2,3]
The above array of integers “numbers_array” is now accessible and editable from the Godot editor.
Exporting NodePaths
A common use case for export is to expose NodePaths. NodePath can be used to store a path to a node. Check out the following code:
export(NodePath) var path_to_node
In this code, “path_to_node” is a NodePath variable that can be used to retrieve or set the path to a node in the scene tree directly from the editor.
Further Exploration with GDScript Export
We have already seen the basic usage of the export keyword with different types of variables. Let’s dig deeper and look at a few more examples which demonstrate other possible applications of this keyword in GDScript scripting.
In addition to exporting plain variable types and arrays, it is also possible to use the export keyword with dictionaries in GDScript, as shown in this code snippet:
export(Dictionary) var hero_stats = {"strength": 10, "dexterity": 8, "constitution": 12}
In this code, “hero_stats” is a dictionary that holds different stats of a hero character in a game. The dictionary can be edited from the editor due to the use of the export keyword.
Furthermore, the export keyword can also be used with custom types defined in GDScript. Consider the following example:
class CharacterStats: var strength var dexterity var constitution export(CharacterStats) var hero_stats = CharacterStats.new()
In this example, we have defined a custom type with the class keyword, and then used the export keyword to make an instance of this class editable in the editor.
The export keyword also allows us to limit the values a variable can take with the enum keyword. See the following example:
enum GameDifficulty {EASY, MEDIUM, HARD} export(GameDifficulty) var difficulty = GameDifficulty.EASY
This code defines an enumeration GameDifficulty with the enum keyword, and the variable “difficulty” can be set to one of these values from the editor.
Lastly, the resource types provided by Godot, such as Textures and Sounds, can be exported too. This makes it straightforward to assign graphical assets or sound files to properties of a game object from the editor. Here is an example:
export(Texture) var sprite_texture
The variable “sprite_texture” is of Texture type and can be assigned a texture resource directly from the editor.
These examples demonstrate the versatility and functionality of the export keyword in GDScript, making it an invaluable tool in your Godot Engine toolkit.
Deep Dive into GDScript Export Functionality
Extending the versatility, GDScript also allows the export keyword to be combined with the onready keyword. Each time a Node is ready, it utilizes the value that was assigned to the variable in the editor. Below is an example:
export var health = 100 onready var max_health = health
In this example, the “max_health” variable will get the value of “health” that was set in the editor, every time a Node is ready.
Along similar lines, the export keyword can be used with setget functions. In this way, a function will be called every time a variable is assigned a value in the editor. See the following example:
export var health setget set_health func set_health(value): health = value print("Health set to ", value)
In the code above, the function set_health is invoked every time “health” is assigned a value in the editor. This provides a platform to execute some additional logic whenever a value is set in the editor.
Going further, GDScript also allows you to export variables of built-in types, like Vector2, Vector3, Color, etc. This aids in adjusting game settings and properties directly through the Godot editor. For example:
export(Vector2) var speed = Vector2(100, 200) export(Color) var sprite_color = Color(1, 1, 1, 1)
In this snippet, a Vector2 variable “speed” and a Color variable “sprite_color” have their default values set that can be changed directly from the editor.
An even more advanced usage of the export keyword is in conjunction with other nodes in the scene tree. This facilitates modifying instances of in-game objects, aiding in a streamlined level design and game mechanics realization. See a common usage:
export(NodePath) var target_node_path var target_node func _ready(): target_node = get_node(target_node_path)
The above snippet shows a NodePath variable being exported. In the editor, we can set the path to another Node. The “_ready” function retrieves the target node using this path.
All these examples enhance the potential of the export keyword, emphasizing its significant role in the process of game development in Godot Engine.</p
Your Learning Journey Continues
Now that you’ve had a glimpse into the power of GDScript Export, the possibilities are endless! The growing world of game development continues to excite and inspire countless careers and creatives. To keep nurturing your passion and skills, we at Zenva provide a wide range of programming and game development courses, suitable for beginners transitioning to professionals.
We invite you to explore our Godot Courses for more detailed learning. Ideal for those who have tasted the basics and are keen to delve further into the fascinating universe of Godot and GDScript.
And for an encompassing learning adventure, consider our Godot Game Development Mini-Degree. A comprehensive compilation of courses designed to instill you with the knowledge to build cross-platform games using Godot 4. From 2D and 3D assets to gameplay control flow, combat systems, and more – this program presents an opportunity to create a sparkling portfolio of real game projects. The courses are accessible, interactive, and indeed a worthy step in your career in game development.
Remember, each module offers completion certificates, adding value to your professional trajectory. With Zenva, you can truly go from beginner to professional. Happy coding!
Conclusion
The game development journey is full of countless exciting opportunities, and mastering Godot and GDScript is one of them. Our Godot Courses deliver industry-standard modules that will propel your game making skills, equipped with all the essential knowledge to take on the real-world challenges in game development with Godot.
At Zenva, we strive to make learning accessible, empowering you with practical knowledge that you can apply immediately. Embrace the adventure ahead in-game development, and let’s create captivating games together! Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
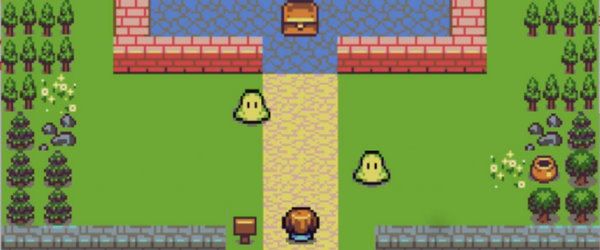
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.