Welcome to the world of game development and coding! Today, we’ll delve into a crucial aspect of scripting in the popular game engine, Godot. This article focuses on understanding and utilizing GDScript’s string concatenation.
If you’re new to Godot or GDScript, fret not! This tutorial is designed in such a way that it is beginner-friendly yet invaluable to the pros. Regardless of your familiarity with programming languages, you will find this piece enlightening and useful.
Table of contents
What is GDScript’s String Concatenation?
Just like any other programming language, GDScript, which is a high-level, dynamically-typed programming language used to create content in the Godot engine, makes use of string concatenation. It is a method that links or merges two or more strings.
Learning how to concatenate strings is pivotal as it helps in setting up dialogues in your game, displaying instructions, or even for debugging purposes.
Why Should I Learn It?
String concatenation might not seem glamorous, but mastering it can add a whole new level of nuance and polish to your games. Proper use of text can improve the user interface, provide players with vital instructions, or even help tell an engaging story.
Moreover, understanding string concatenation in GDScript will enable you to transfer that knowledge to other languages as most programming formats have a version of this fundamental concept.
Basic Syntax for String Concatenation
GDScript uses the ‘+’ operator to concatenate or link two strings. This is a straightforward way to concatenate, and you’ve probably used something similar in other languages.
Here’s an extremely simple example:
var string1 = "Hello, " var string2 = "world!" print(string1 + string2) # Prints: Hello, world!
In this example, the GDScript print() function outputs the result of string1 + string2, which is “Hello, world!”. Making use of the ‘+’, we effectively merged the two strings.
Concatenating Variables and Strings
Often in game development, you’ll want to merge not only two strings but also a mix of variables and strings. This is where GDScript’s flexibility shines.
Consider this example:
var player_name = "Zenva" var level = 9 print("Player: " + player_name + " is at Level: " + str(level)) # Prints: Player: Zenva is at Level: 9
In this snippet, GDScript concatenates the player_name variable (a string), the level variable (an integer), and a few other strings. Notice how we used the built-in str() function to convert the integer level to a string before concatenating.
Concatenating with the str() Function
You may require to concatenate a string with a non-string type like a number or a boolean in certain circumstances. That’s when the str() function becomes useful.
Here’s an example:
var numberOfEnemies = 10 print("Number of enemies: " + str(numberOfEnemies)) # Prints: Number of enemies: 10
In the above example, the str() function converts the integer numberOfEnemies to a string so that it can be concatenated with the other string.
Multiline String Concatenation
GDScript allows multiline string concatenation using three double quotes(“””). This can be especially useful for dialogue design or player instructions.
Check out this useful feature:
var multilineString = """ Welcome to Zenva Academy, The best place to <a class="wpil_keyword_link" href="https://gamedevacademy.org/how-to-learn-code-beginners-coding/" target="_blank" rel="noopener" title="learn coding" data-wpil-keyword-link="linked">learn coding</a> and game creation! """ print(multilineString)
The output here will be exactly as it appears within the triple quotes, spacing and line breaks included!
Using the String Format Method in GDScript
Apart from using the ‘+’ operator, GDScript also provides the format() function for string concatenation. This feature is particularly useful when working with multiple variables.
Here’s an example demonstrating this:
var player_name = "Zenva" var level = 9 print("Player: {0} is at Level: {1}".format(player_name, level)) # Prints: Player: Zenva is at Level: 9
In the format expression, the curly braces {} are placeholders. The format() function replaces these placeholders with its respective arguments in order, producing a neat and efficient string concatenation.
Direct String Conversion on Initialization
If you are sure a variable will be used as a string, you can directly initialize it as a string. Look at this example:
var score = str(100) print("Your score is: " + score) # Prints: Your score is: 100
We have declared the score variable as a string during initialization itself. Therefore, there is no need for further conversion later during concatenation.
Precisely, you save a few keystrokes this way!
Replacing Parts of a String
With GDScript, you can replace certain parts of a string by using the replace() method. This approach is handy for many scenarios such as correcting typos, changing names, or adjusting messages dynamically.
var welcomeMessage = "Hello, Zenba Player!" welcomeMessage = welcomeMessage.replace("Zenba", "Zenva") print(welcomeMessage) # Prints: Hello, Zenva Player!
Our typo was “Zenba”, and we replaced it with “Zenva”. The replace() function takes two parameters. The first is the string that we aim to replace, and the second is the string that we want to substitute in.
F-Strings in GDScript
Another feature in GDScript’s string concatenation toolset is the F-string format.
var player_name = "Zenva" var level = 9 print(f"Player: {player_name} is at Level: {level}") # Prints: Player: Zenva is at Level: 9
Simply appending ‘f’ before the string allows you to use the curly brace {} placeholder format directly within the string. Just like Python, GDScript will replace these placeholders with the named variable’s value, leading to convenient and readable code.
Congratulations on grasping the crucial concept of String Concatenation in GDScript, which will indubitably be a vital tool in your Godot engine journey!
Advancing with String Manipulation in GDScript
As you’re getting comfortable with string concatenation, let’s work on some complex yet interesting examples and explore other aspects of string manipulation in GDScript. These advanced concepts will be extremely beneficial in creating high-quality, dynamic content for your games.
Using Placeholders in String Formatting
When dealing with dynamic values, placeholders in a string come in handy. Here is an example using the ‘%’ symbol as the placeholder:
var player_name = "Zenva" var level = 9 print("Player: %s is at Level: %d" % [player_name, level]) # Prints: Player: Zenva is at Level: 9
‘%s’ is the place holder for a string, while ‘%d’ is the placeholder for integers. And following the string, we have a ‘%’ symbol and an array of values. The format of these placeholders will be decided by the datatype of the variables in the array.
Using the to_upper() Function
GDScript’s to_upper() function allows you to change all the characters in a string to uppercase:
var lowercase_string = "zenva academy" var uppercase_string = lowercase_string.to_upper() print(uppercase_string) # Prints: ZENVA ACADEMY
Using the to_lower() Function
On the contrary, the to_lower() function changes all characters in a string to lowercase:
var uppercase_string = "ZENVA ACADEMY" var lowercase_string = uppercase_string.to_lower() print(lowercase_string) # Prints: zenva academy
Concatenating Using sprintf() Function
Similar to the example we saw with placeholders above, the sprintf() function is another way to concatenate strings with variables:
var health = 70 print("Health: " + sprintf("%d%%", health)) # Prints: Health: 70%
In this example, we are printing the health of a character where the ‘%’ character itself needs to be included in the string. GDScript handles this smoothly using the sprintf() function.
Clearly, GDScript’s approach to string concatenation is versatile, accommodating almost any use case in an elegant, readable way. By getting comfortable with these concepts, you’ll be well-equipped to handle text-based tasks in your Godot engine projects!
At Zenva, we believe that these string manipulation techniques are not just powerful but integral to creating immersive and interactive gaming experiences. So go ahead and experiment with these snippets in your own Godot projects!
Where to Go Next?
As we’ve unveiled the power of string concatenation in GDScript together, you might be wondering, “What’s next on this exciting journey of game development?”
The answer? Dive deep and broaden your understanding of Godot and GDScript. If this tutorial was engaging and helpful to you, we encourage you to check out our Godot Game Development Mini-Degree. This comprehensive collection of courses will take you through the journey of building cross-platform games using the Godot engine. From using 2D and 3D assets, mastering GDScript, understanding gameplay control flow, to creating various game mechanics, the mini-degree leaves no stone unturned.
Don’t limit yourself to just one course; we have an entire suite of Godot Courses to satisfy your curiosity and elevate your game development skills. With these courses, not only will you learn coding and create games, but you will also move one step closer to becoming a game development professional!
Conclusion
The world of game development is intriguing and exciting, and mastering GDScript’s potent string concatenation and manipulation is a vital brick in the building of your game creation journey. Crafting text in your games is much more than arranging a few letters on the screen – it’s what enriches a player’s experience, propels narrative, and makes your creation memorable.
The Godot engine and GDScript throw open doors of endless possibilities. As you continue learning and exploring, let Zenva be your faithful guide and mentor. Our Godot Game Development Mini-Degree is waiting to take you to greater heights in game creation. Together, let’s create and enjoy the art of awesome game development!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
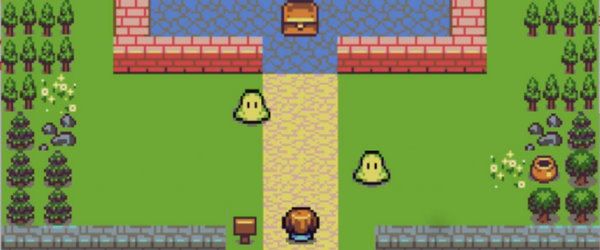
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.