Welcome to a comprehensive tutorial where today we’ll be delving into the world of Boolean programming. Whether you’re just beginning your coding adventure or you’re a seasoned programmer brushing up on fundamentals, Boolean logic is a pillar of programming that you’ll encounter in nearly all facets of development. By understanding the binary simplicity of true and false, you can construct complex logic in games, data analysis, and more. Now, let’s unwrap the essentials of Boolean programming and discover why it’s a cornerstone of coding logic.
Table of contents
What is Boolean Programming?
At the heart of every decision-making process in programming lies a simple, yet powerful concept: Boolean logic. This type of programming hinges on the idea that all values can be reduced to either true or false—named after George Boole, a mathematician who laid the groundwork for this binary system. Booleans are the building blocks for creating conditions and flow control in code, influencing how a program behaves in response to different inputs.
What is it for?
Boolean programming comes into play when we need to make decisions in our code. From checking if a player has enough points to level up in a game to validating user input in a web form, Booleans help us execute specific actions based on conditions. This decision-making ability is crucial in developing dynamic and responsive applications.
Why Should I Learn It?
Efficiency and Clarity: Boolean logic allows you to write clear and concise code, making your programs more efficient and easier to understand.
Universal Concept: Boolean logic is supported by virtually all programming languages, making it a universal concept that boosts your versatility as a programmer.
Foundational Knowledge: Grasping Boolean logic is essential for advancing in programming, as it lays the foundation for more complex concepts like algorithms and data structures.
By getting to grips with Boolean programming, you are laying a solid foundation for your future projects. With a firm handle on this topic, you can write more effective code and enhance your problem-solving skills. Now, let’s take our first steps into the world of Boolean programming with some coding examples!
Understanding Boolean Values and Expressions
Boolean values are the simplest form of data; they can be either true or false. These values are resultant of Boolean expressions, which are conditions that evaluate to a Boolean value. Let’s start by defining Boolean values in various programming languages:
// In JavaScript var isGameOver = false; // In Python is_game_over = False // In C# bool isGameOver = false;
A Boolean expression can be a direct comparison between variables or values. Here are some basic comparisons:
// In JavaScript var score = 100; var isWinner = score >= 50; // Evaluates to true // In Python score = 100 is_winner = score >= 50 # Evaluates to true // In C# int score = 100; bool isWinner = score >= 50; // Evaluates to true
Conditional Statements Using Booleans
Conditional statements form the backbone of decision making in programming. We use if-else structures to perform different actions based on the truth value of Boolean expressions:
// In JavaScript if (isGameOver) { console.log("Game Over!"); } else { console.log("Keep playing!"); } // In Python if is_game_over: print("Game Over!") else: print("Keep playing!") // In C# if (isGameOver) { Console.WriteLine("Game Over!"); } else { Console.WriteLine("Keep playing!"); }
Boolean values can also be manipulated with logical operators like AND (&& or and), OR (|| or or), and NOT (! or not).
// In JavaScript var hasLives = true; var hasTimeLeft = false; if (hasLives && hasTimeLeft) { console.log("Game continues!"); } else { console.log("Game over due to a condition!"); } // In Python has_lives = True has_time_left = False if has_lives and has_time_left: print("Game continues!") else: print("Game over due to a condition!") // In C# bool hasLives = true; bool hasTimeLeft = false; if (hasLives && hasTimeLeft) { Console.WriteLine("Game continues!"); } else { Console.WriteLine("Game over due to a condition!"); }
Using Booleans in Loops
Loops often rely on Boolean values to determine when they should start or stop. Here’s an example using a while loop that continues until a Boolean condition is no longer true:
// In JavaScript var keepPlaying = true; while (keepPlaying) { // Code for playing the game // An event occurs that sets keepPlaying to false } // In Python keep_playing = True while keep_playing: # Code for playing the game # An event occurs that sets keep_playing to False // In C# bool keepPlaying = true; while (keepPlaying) { // Code for playing the game // An event occurs that sets keepPlaying to false }
Switching Boolean values is also a frequent operation, such as toggling a flag in response to an event:
// In JavaScript var isPaused = false; function togglePause() { isPaused = !isPaused; } // In Python is_paused = False def toggle_pause(): global is_paused is_paused = not is_paused // In C# bool isPaused = false; void TogglePause() { isPaused = !isPaused; }
With these basics in hand, you’re on your way to masterfully controlling the logic flow within your programs. Remember, Boolean programming is not just about ‘true’ or ‘false’; it’s about understanding the conditions that lead to these states and utilizing them to drive your application’s behavior.
Let’s dive deeper into Boolean programming by exploring a range of different applications and more complex logic constructs. Through these examples, you’ll see the versatility of Boolean logic in action within various coding scenarios.
Complex Conditions with Boolean Logic
Understanding how to combine Boolean expressions using logical operators is vital for building complex conditions. Here are some examples that illustrate how we might use the AND, OR, and NOT operators together:
// In JavaScript var hasPermission = true; var isAdmin = false; if (hasPermission || isAdmin) { console.log("Access granted"); } else { console.log("Access denied"); } // In Python has_permission = True is_admin = False if has_permission or is_admin: print("Access granted") else: print("Access denied") // In C# bool hasPermission = true; bool isAdmin = false; if (hasPermission || isAdmin) { Console.WriteLine("Access granted"); } else { Console.WriteLine("Access denied"); }
Handling multiple complex conditions can be streamlined by encapsulating them into Boolean variables, which makes the if-else branching cleaner and more readable:
// In JavaScript var isAtEndGame = true; var hasAllItems = false; var canAccessFinalLevel = isAtEndGame && hasAllItems; if (canAccessFinalLevel) { console.log("Welcome to the final level!"); } else { console.log("You must gather all the items first."); } // In Python is_at_end_game = True has_all_items = False can_access_final_level = is_at_end_game and has_all_items if can_access_final_level: print("Welcome to the final level!") else: print("You must gather all the items first.") // In C# bool isAtEndGame = true; bool hasAllItems = false; bool canAccessFinalLevel = isAtEndGame && hasAllItems; if (canAccessFinalLevel) { Console.WriteLine("Welcome to the final level!"); } else { Console.WriteLine("You must gather all the items first."); }
Using Booleans for Error Checking
Booleans are often used to check for errors or invalid conditions. For instance, after calling a function that could fail, we might check a Boolean flag to see if we need to handle an error:
// In JavaScript function loadGame(data) { // Attempt to load game, returns true on success } var loadSuccess = loadGame(gameData); if (!loadSuccess) { console.error("Failed to load game!"); } // In Python def load_game(data): # Attempt to load game, returns True on success return True load_success = load_game(game_data) if not load_success: print("Failed to load game!") // In C# bool LoadGame(object data) { // Attempt to load game, returns true on success } bool loadSuccess = LoadGame(gameData); if (!loadSuccess) { Console.Error.WriteLine("Failed to load game!"); }
Toggling States in Games
Booleans are also incredibly handy for toggling states in games. For example, we often have to control whether a menu or a setting is active:
// In JavaScript var menuOpen = false; function toggleMenu() { menuOpen = !menuOpen; console.log(menuOpen ? "Menu Opened" : "Menu Closed"); } // In Python menu_open = False def toggle_menu(): global menu_open menu_open = not menu_open print("Menu Opened" if menu_open else "Menu Closed") // In C# bool menuOpen = false; void ToggleMenu() { menuOpen = !menuOpen; Console.WriteLine(menuOpen ? "Menu Opened" : "Menu Closed"); }
Learning to utilize Booleans for such toggling operations can significantly simplify the state management in your code, contributing to a cleaner design and more straightforward logic. Whether you’re managing game states, user preferences, or display settings, mastering Boolean operations will empower you to create intuitive functionalities for your users.
We hope these examples have proven the vast utilities of Boolean programming. By incorporating these simple yet powerful constructs, you’re effectively adding control and precision to your code, an essential skill set on your journey as a developer. Keep practicing with Booleans, and you’ll soon uncover even more ways they can cater to the logic of your unique coding ventures!
Let’s explore additional ways Boolean values can enhance our programming with various real-world code examples. As we progress, pay close attention to the simplicity and elegance that Boolean logic infuses into our coding solutions.
In the realm of game development, Booleans often control gameplay mechanics. Below is an example where a simple Boolean value dictates whether a player can attack:
// In JavaScript var canAttack = true; function attack() { if (canAttack) { console.log("Player attacks!"); canAttack = false; // Assuming there's a cooldown } } // In Python can_attack = True def attack(): global can_attack if can_attack: print("Player attacks!") can_attack = False # Assuming there's a cooldown // In C# bool canAttack = true; void Attack() { if (canAttack) { Console.WriteLine("Player attacks!"); canAttack = false; // Assuming there's a cooldown } }
Similarly, we use Booleans to manage game object visibility. In this scenario, we have a Boolean controlling the visibility of an object, like an enemy on a radar:
// In JavaScript var isVisible = false; function toggleVisibility() { isVisible = !isVisible; console.log(isVisible ? "Enemy is visible." : "Enemy is hidden."); } // In Python is_visible = False def toggle_visibility(): global is_visible is_visible = not is_visible print("Enemy is visible." if is_visible else "Enemy is hidden.") // In C# bool isVisible = false; void ToggleVisibility() { isVisible = !isVisible; Console.WriteLine(isVisible ? "Enemy is visible." : "Enemy is hidden."); }
Another frequent use of Booleans is in form validations. When submitting a form, we need to check if all fields meet certain criteria. Booleans help us track the validity of each field:
// In JavaScript var isNameValid = false; var isEmailValid = false; function validateForm() { // Pretend we validate each field and set the boolean accordingly isNameValid = // Some validation code for name isEmailValid = // Some validation code for email if (isNameValid && isEmailValid) { console.log("Form is valid and ready to submit!"); } else { console.log("Form is invalid. Please check the input fields."); } } // In Python is_name_valid = False is_email_valid = False def validate_form(): # Pretend we validate each field and set the boolean accordingly is_name_valid = # Some validation code for name is_email_valid = # Some validation code for email if is_name_valid and is_email_valid: print("Form is valid and ready to submit!") else: print("Form is invalid. Please check the input fields.") // In C# bool isNameValid = false; bool isEmailValid = false; void ValidateForm() { // Pretend we validate each field and set the boolean accordingly isNameValid = // Some validation code for name isEmailValid = // Some validation code for email if (isNameValid && isEmailValid) { Console.WriteLine("Form is valid and ready to submit!"); } else { Console.WriteLine("Form is invalid. Please check the input fields."); } }
When it comes to security features, such as a simple login system, Booleans are used to keep track of authentication status:
// In JavaScript var isAuthenticated = false; function login(username, password) { isAuthenticated = username === "user" && password === "pass"; // Simple check console.log(isAuthenticated ? "Login successful." : "Login failed."); } // In Python is_authenticated = False def login(username, password): global is_authenticated is_authenticated = username == "user" and password == "pass" # Simple check print("Login successful." if is_authenticated else "Login failed.") // In C# bool isAuthenticated = false; void Login(string username, string password) { isAuthenticated = username == "user" && password == "pass"; // Simple check Console.WriteLine(isAuthenticated ? "Login successful." : "Login failed."); }
In interactive web pages or applications, Booleans are used to manage the state of menus, panels, and interactive elements. Consider a sidebar that users can expand or collapse:
// In JavaScript var isSidebarOpen = false; function toggleSidebar() { isSidebarOpen = !isSidebarOpen; // Code to visually expand or collapse the sidebar goes here } // In Python # Note: Since Python is not commonly used for client-side web programming, # the example would be different for a server-side Python web framework like Django or Flask. // In C# // Assuming a C# ASP.NET MVC project where the controller can influence view behavior bool isSidebarOpen = false; void ToggleSidebar() { isSidebarOpen = !isSidebarOpen; // Code that impacts the view's rendering of the sidebar }
As we’ve explored, Boolean programming is a versatile and integral aspect of coding, used for controlling flow, checking conditions, managing states, and more. These simple true/false values can indeed build the complex constructs that make modern applications function. To become more proficient, continue experimenting with Booleans in various scenarios and appreciate their capability to simplify and streamline the logic in your code. Remember, every sophisticated program begins with understanding the basics, and Boolean programming is as fundamental as it gets!
Further Your Programming Journey with Zenva
You’ve laid the groundwork with Boolean programming, grasped the basics, and explored how simple true-false logic can drive complex applications. Now, it’s time to take your coding skills to the next level. Embrace the challenge to continue learning and growing in your coding journey with us at Zenva!
Dive deep into the world of Python, one of the most versatile and widely-used programming languages, with our Python Mini-Degree. This comprehensive collection of courses is crafted to guide you from the fundamentals to more advanced topics, allowing you to build practical projects and cement your knowledge. Whether you’re aspiring to delve into game development, create dynamic applications, or automate tasks, our Python Mini-Degree is a valuable resource.
For those eager to explore even broader programming horizons, check out our full range of Programming courses. Across over 250 supported courses, beginners can embark on a new career path while seasoned programmers can keep their skills sharp and up-to-date. No matter where you are in your coding journey, Zenva is here to help you reach new heights. So, keep the momentum going – your future in programming awaits!
Conclusion
Booleans may be the simplest data type you’ll work with in programming, but as you’ve seen, they are incredibly powerful. Entire systems are built upon the binary decisions these true and false values provide. We at Zenva hope that this insight into Boolean programming has sparked an even greater interest in the world of coding. As you continue to learn and build, remember that each line of code is a step on your journey to becoming an adept coder.
Don’t let your learning stop here. With Zenva’s comprehensive Python Mini-Degree, you can reinforce your newfound knowledge and expand upon it, crafting your very own digital worlds and innovative applications. The realm of code is vast and full of possibilities – let’s continue exploring it together and turn your programming aspirations into reality. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
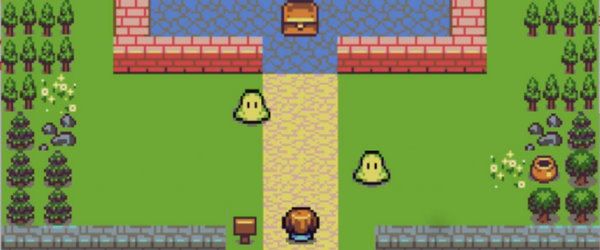
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.